Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial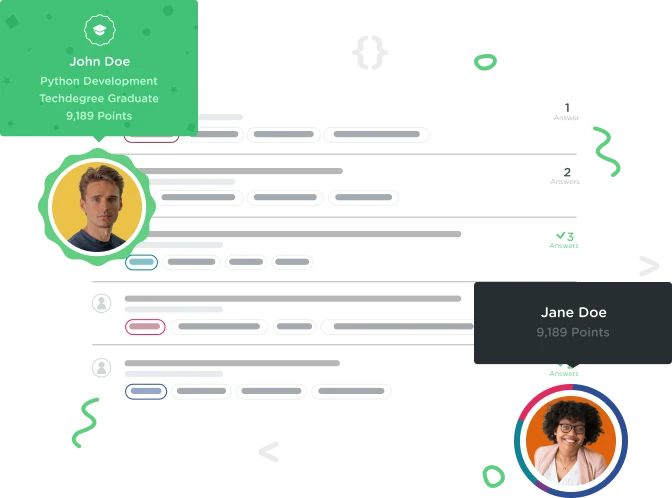

Monis bana
61 PointsApp crashers when Hourly button is clicked
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import com.mab.stormy.R;
import com.mab.stormy.weather.Hour;
/**
* Created by montur on 9/15/2016.
*/
public class HourAdapter extends RecyclerView.Adapter <HourAdapter.HourViewHolder>{
public Hour[] mHours;
public HourAdapter(Hour[] hours){
mHours=hours;
}
@Override
public HourViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.hourly_list_item,parent,false);
HourViewHolder ViewHolder =new HourViewHolder(view);
return ViewHolder;
}
@Override
public void onBindViewHolder(HourViewHolder holder, int position) {
holder.bindHour(mHours[position]);
}
@Override
public int getItemCount() {
return mHours.length;
}
public class HourViewHolder extends RecyclerView.ViewHolder {
public TextView mTimeLabel;
public TextView mSummaryLabel;
public TextView mTemperatureLabel;
public ImageView mIconImageView;
public HourViewHolder(View itemView) {
super(itemView);
mTimeLabel=(TextView)itemView.findViewById(R.id.timeLabel);
mSummaryLabel=(TextView)itemView.findViewById(R.id.summaryLabel);
mTemperatureLabel=(TextView)itemView.findViewById(R.id.temperatureLabel);
mIconImageView=(ImageView)itemView.findViewById(R.id.iconImageView);
}
public void bindHour(Hour hour){
mTimeLabel.setText(hour.getHour());
mSummaryLabel.setText(hour.getSummary());
mTemperatureLabel.setText(hour.getTemperature());
mIconImageView.setImageResource(hour.getIconId());
}
}
}
//Error
FATAL EXCEPTION: main
Process: com.mab.stormy, PID: 24299
android.content.res.Resources$NotFoundException: String resource ID #0x4f
at android.content.res.Resources.getText(Resources.java:312)
at android.support.v7.widget.ResourcesWrapper.getText(ResourcesWrapper.java:52)
at android.widget.TextView.setText(TextView.java:4427)
at com.mab.stormy.adapters.HourAdapter$HourViewHolder.bindHour(HourAdapter.java:55)
at com.mab.stormy.adapters.HourAdapter.onBindViewHolder(HourAdapter.java:30)
at com.mab.stormy.adapters.HourAdapter.onBindViewHolder(HourAdapter.java:16)
at android.support.v7.widget.RecyclerView$Adapter.onBindViewHolder(RecyclerView.java:5746)
at android.support.v7.widget.RecyclerView$Adapter.bindViewHolder(RecyclerView.java:5779)
at android.support.v7.widget.RecyclerView$Recycler.getViewForPosition(RecyclerView.java:5016)
at android.support.v7.widget.RecyclerView$Recycler.getViewForPosition(RecyclerView.java:4892)
at android.support.v7.widget.LinearLayoutManager$LayoutState.next(LinearLayoutManager.java:2029)
at android.support.v7.widget.LinearLayoutManager.layoutChunk(LinearLayoutManager.java:1414)
at android.support.v7.widget.LinearLayoutManager.fill(LinearLayoutManager.java:1377)
at android.support.v7.widget.LinearLayoutManager.onLayoutChildren(LinearLayoutManager.java:578)
at android.support.v7.widget.RecyclerView.dispatchLayoutStep2(RecyclerView.java:3239)
at android.support.v7.widget.RecyclerView.dispatchLayout(RecyclerView.java:3048)
at android.support.v7.widget.RecyclerView.onLayout(RecyclerView.java:3497)
at android.view.View.layout(View.java:16651)
at android.view.ViewGroup.layout(ViewGroup.java:5440)
at android.widget.RelativeLayout.onLayout(RelativeLayout.java:1079)
at android.view.View.layout(View.java:16651)
at android.view.ViewGroup.layout(ViewGroup.java:5440)
at android.widget.FrameLayout.layoutChildren(FrameLayout.java:336)
at android.widget.FrameLayout.onLayout(FrameLayout.java:273)
at android.view.View.layout(View.java:16651)
at android.view.ViewGroup.layout(ViewGroup.java:5440)
at android.widget.LinearLayout.setChildFrame(LinearLayout.java:1743)
at android.widget.LinearLayout.layoutVertical(LinearLayout.java:1586)
at android.widget.LinearLayout.onLayout(LinearLayout.java:1495)
at android.view.View.layout(View.java:16651)
at android.view.ViewGroup.layout(ViewGroup.java:5440)
at android.widget.FrameLayout.layoutChildren(FrameLayout.java:336)
at android.widget.FrameLayout.onLayout(FrameLayout.java:273)
at android.view.View.layout(View.java:16651)
at android.view.ViewGroup.layout(ViewGroup.java:5440)
at android.widget.LinearLayout.setChildFrame(LinearLayout.java:1743)
at android.widget.LinearLayout.layoutVertical(LinearLayout.java:1586)
at android.widget.LinearLayout.onLayout(LinearLayout.java:1495)
at android.view.View.layout(View.java:16651)
at android.view.ViewGroup.layout(ViewGroup.java:5440)
at android.widget.FrameLayout.layoutChildren(FrameLayout.java:336)
at android.widget.FrameLayout.onLayout(FrameLayout.java:273)
at com.android.internal.policy.PhoneWindow$DecorView.onLayout(PhoneWindow.java:2678)
at android.view.View.layout(View.java:16651)
at android.view.ViewGroup.layout(ViewGroup.java:5440)
at android.view.ViewRootImpl.performLayout(ViewRootImpl.java:2183)
at android.view.ViewRootImpl.performTraversals(ViewRootImpl.java:1943)
at android.view.ViewRootImpl.doTraversal(ViewRootImpl.java:1119)
at android.view.ViewRootImpl$TraversalRunnable.run(ViewRootImpl.java:6060)
at android.view.Choreographer$CallbackRecord.run(Choreographer.java:858)
at android.view.Choreographer.doCallbacks(Choreographer.java:670)
at android.view.Choreographer.doFrame(Choreographer.java:606)
at android.view.Choreographer$FrameDisplayEventReceiver.run(Choreographer.java:844)
at android.os.Handler.handleCallback(Handler.java:746)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5443)
at java.lang.reflect.Method.invoke(Native
Edited For Readability - Dane E. Parchment Jr. (moderator)
2 Answers

Seth Kroger
56,413 PointsOne of Hour's values is returning an integer, getHour() if I counted the lines right. getText() interprets a integer argument as a resource id, not a number to convert into a string. You'll need to do that yourself by one of the usual methods: smushing ( + ""), String.format(), Integer.toString(), etc.
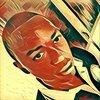
kobbyberks
10,722 PointsThe getTemperature()
method returns an Integer and setText
is only used for Strings, so you need to convert the temperature value to a String like so:
mTemperatureLabel.setText(hour.getTemperature() + "");