Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial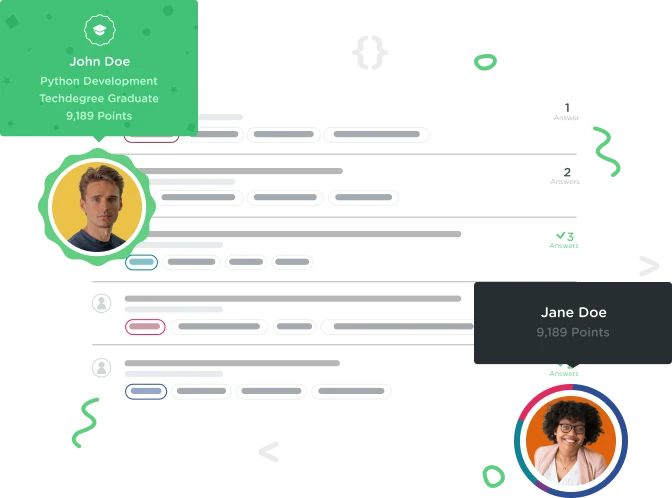

Mustafa Alordowny
4,495 Pointsapp crashes
app crashes when i run it because of this line runOnUiThread(new Runnable() { @Override public void run() { mProgressBar.setVisibility(View.INVISIBLE); } });
HERE IS THE CODE:
package com.musmost.stormy;
import android.content.Context; import android.graphics.drawable.Drawable; import android.net.ConnectivityManager; import android.net.NetworkInfo; import android.support.v4.app.ActivityCompat; import android.support.v4.content.ContextCompat; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.ProgressBar; import android.widget.TextView; import android.widget.ImageView; import android.widget.Toast;
import com.google.android.gms.common.api.GoogleApiClient; import com.google.android.gms.location.LocationServices;
import org.json.JSONException; import org.json.JSONObject; import org.w3c.dom.Text;
import java.io.IOException;
import butterknife.BindView; import butterknife.ButterKnife; import okhttp3.Call; import okhttp3.Callback; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response;
public class MainActivity extends AppCompatActivity { public static final String TAG = MainActivity.class.getSimpleName();
private ImageView mRefresh;
private TextView mTemperatureLabel;
private TextView mTimeLabel;
private TextView mHumidityValue;
private TextView mPrecipValue;
private TextView mSummaryLabel;
private ImageView mIconImageView;
private ProgressBar mProgressBar;
private CurrentWeather mCurrentWeather;
private Double mLatitude = 37.8267;
private Double mLongitude = -122.4233;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mTemperatureLabel = (TextView)findViewById(R.id.tempratureLabel) ;
mTimeLabel = (TextView)findViewById(R.id.timeText);
mProgressBar = (ProgressBar) findViewById(R.id.progressBar);
runOnUiThread(new Runnable() {
@Override
public void run() {
mProgressBar.setVisibility(View.INVISIBLE);
}
});
mHumidityValue =(TextView) findViewById(R.id.humidityValue);
mPrecipValue = (TextView) findViewById(R.id.rainValue);
mSummaryLabel = (TextView) findViewById(R.id.summaryLabel);
mIconImageView =(ImageView) findViewById(R.id.icon);
mRefresh = (ImageView)findViewById(R.id.refreshButton);
mRefresh.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
getForecast(mLatitude,mLongitude);
}
});
getForecast(mLatitude,mLongitude);
}
private void getForecast(Double latitude,Double longitude) {
String secretKey = "e0acaf63d7ab893caba31a989bdeea36";
String darkSkyUrl = "https://api.darksky.net/forecast/" + secretKey + "/" + latitude + "," + longitude;
if (isNetworkAvailable()) {
toggleProgressBar();
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(darkSkyUrl).build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData = response.body().string();
if (response.isSuccessful()) {
Log.v(TAG, jsonData);
mCurrentWeather = getCurrentDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
setWeatherDeatils();
toggleProgressBar();
}
});
} else {
alertUser();
}
} catch (IOException | JSONException e) {
Log.e(TAG, "Exception Caught: ", e);
}
}
});
} else {
AlertDialogFragment fragment = new AlertDialogFragment(getString(R.string.no_network_message));
fragment.show(getFragmentManager(), "network error");
}
}
private void toggleProgressBar() {
runOnUiThread(new Runnable() {
@Override
public void run() {
if(mProgressBar.getVisibility() == View.INVISIBLE) {
mProgressBar.setVisibility(View.VISIBLE);
mRefresh.setVisibility(View.INVISIBLE);
} else {
mProgressBar.setVisibility(View.INVISIBLE);
mRefresh.setVisibility(View.VISIBLE);
}
}
});
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setTemprature(currently.getDouble("temperature"));
currentWeather.setSummary(currently.getString("summary"));
// currentWeather.setTimezone(currently.getString("timezone"));
Log.d(TAG,currentWeather.getFormattedTime());
return currentWeather;
}
private void setWeatherDeatils() {
mTimeLabel.setText("At " + mCurrentWeather.getFormattedTime() + " it will be");
mTemperatureLabel.setText(mCurrentWeather.getTemprature()+"");
mHumidityValue.setText(mCurrentWeather.getHumidity()+"");
mPrecipValue.setText(mCurrentWeather.getPrecipChance()+"");
mSummaryLabel.setText(mCurrentWeather.getSummary());
Drawable drawable = getResources().getDrawable(mCurrentWeather.getIconId());
mIconImageView.setImageDrawable(drawable);
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alertUser() {
AlertDialogFragment fragment = new AlertDialogFragment(getString(R.string.error_message));
fragment.show(getFragmentManager(), "error connecting");
toggleProgressBar();
}
}
HERE IS THE LOG: --------- beginning of crash 10-27 03:12:03.979 2601-2601/? E/AndroidRuntime: FATAL EXCEPTION: main Process: com.musmost.stormy, PID: 2601 java.lang.RuntimeException: Unable to start activity ComponentInfo{com.musmost.stormy/com.musmost.stormy.MainActivity}: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.ProgressBar.setVisibility(int)' on a null object reference at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2416) at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476) at android.app.ActivityThread.-wrap11(ActivityThread.java) at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344) at android.os.Handler.dispatchMessage(Handler.java:102) at android.os.Looper.loop(Looper.java:148) at android.app.ActivityThread.main(ActivityThread.java:5417) at java.lang.reflect.Method.invoke(Native Method) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616) Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.ProgressBar.setVisibility(int)' on a null object reference at com.musmost.stormy.MainActivity$1.run(MainActivity.java:62) at android.app.Activity.runOnUiThread(Activity.java:5511) at com.musmost.stormy.MainActivity.onCreate(MainActivity.java:59) at android.app.Activity.performCreate(Activity.java:6237) at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1107) at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2369) at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2476) at android.app.ActivityThread.-wrap11(ActivityThread.java) at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1344) at android.os.Handler.dispatchMessage(Handler.java:102) at android.os.Looper.loop(Looper.java:148) at android.app.ActivityThread.main(ActivityThread.java:5417) at java.lang.reflect.Method.invoke(Native Method) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
1 Answer
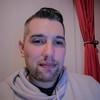
Daniel Hartin
5,311 PointsHi Mustafa,
You are getting a NullPointerException when trying to set the visibilty of the mProgressBar View. There are a number of things that can cause this but checking through your code I suspect that it is simply that you are missing a call to setContentView(R.layout.main) (or whatever your layout file is called) right after the call to the super class in your MainActivity.
Hope this helps Daniel