Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial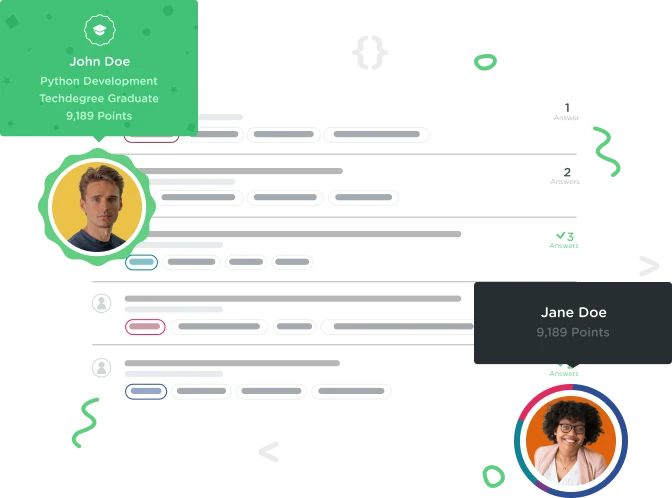

Bobby Varghese
540 PointsApp Crashes After Pushing Button
So I have set the text of all the TextViews and Buttons to "default". What I have coded for is randomly picking a string out of array and setting it equal to on of the TextViews and setting another TextView to the index of the array, but when I push the button, it crashes.

Bobby Varghese
540 Pointspublic class Words { public String [] phrases = { "java", "c++", "python", "javascript", "PHP", "assembly" };
public int num = 0;
public String pickFact ()
{
Random randomizer = new Random();
num = randomizer.nextInt(phrases.length);
String picked = phrases[num];
return picked;
}
public int numPicked ()
{
int i = 0;
while (i != num)
{
i++;
};
return i;
}
}
public class FunFactsActivity extends AppCompatActivity {
private Words mFunFacts = new Words();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
/*Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);*/
//Declare View variables and assign them the Views from the layout file
final TextView factlabel = (TextView) findViewById(R.id.FirstTextView);
Button FactButton = (Button) findViewById(R.id.FactButton);
final TextView titleTextView = (TextView) findViewById(R.id.titleTextView);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v)
{
String fact = mFunFacts.pickFact();
//String factNum = mFunFacts.pickNum() + "";
factlabel.setText(fact);
int num = mFunFacts.numPicked();
titleTextView.setText(num);
}
};
FactButton.setOnClickListener(listener);
// FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
/* fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Snackbar.make(view, "Replace with your own action", Snackbar.LENGTH_LONG)
.setAction("Action", null).show();
}
});*/
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_fun_facts, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
1 Answer

Seth Kroger
56,413 PointsRemember you shouldn't use a number type like int with setText. You need to "smush" it with an empty string first.
titleTextView.setText(num + "");
I should mention that your numPicked() is a little complicated for what is does. All you really need to do is return num;
and that's it.
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsCan you post your code so we can take a look?