Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial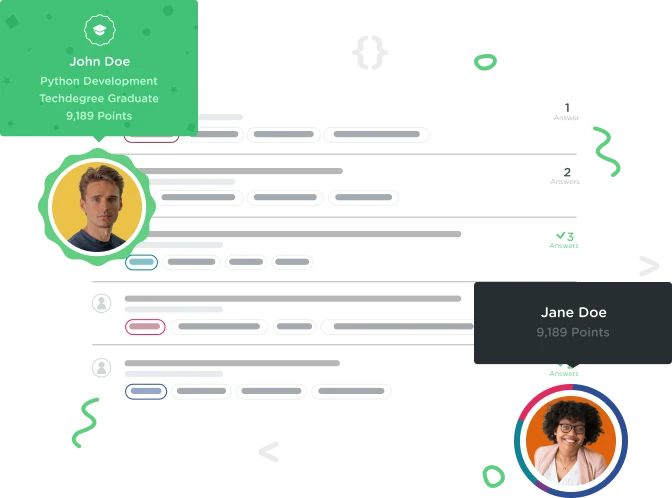
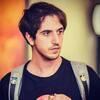
Jonathan Leon
18,813 PointsApp crashes Attempt to invoke virtual method 'android.view.View android.view.Window.findViewById(int)' on a null objec
My thought is that the button doesn't have a value by the time it tries to set a click listener to it, I tried several things but it won't work, the code looks exactly like Ben's :(
package com.wo1v3r.interactivestory;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
private EditText mNameField;
private Button mStartButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mNameField = (EditText) findViewById(R.id.nameEditText);
mStartButton = (Button) findViewById(R.id.startButton);
mStartButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String name = mNameField.getText().toString();
Toast.makeText(MainActivity.this,name,Toast.LENGTH_LONG).show();
}
});
}
}
1 Answer
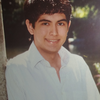
Jon Kussmann
Courses Plus Student 7,254 PointsHi Jonathan,
Your Activity does not know which xml layout file to "search" for to get your Button and EditText. You need to call setContentView()
public class MainActivity extends Activity {
private EditText mNameField;
private Button mStartButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.nameOfYourXMLLayout); // You need to have this line
mNameField = (EditText) findViewById(R.id.nameEditText);
mStartButton = (Button) findViewById(R.id.startButton);
mStartButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String name = mNameField.getText().toString();
Toast.makeText(MainActivity.this,name,Toast.LENGTH_LONG).show();
}
});
}
}
I hope this helps. If not let me know.
Jonathan Leon
18,813 PointsJonathan Leon
18,813 PointsMan I just saw this and facepalmed myself and really hoped to delete the post before someone notices because I'm trying crazy things for an hour already,
Do you have an idea why that line doesn't come through when I make a new project? :|
Jon Kussmann
Courses Plus Student 7,254 PointsJon Kussmann
Courses Plus Student 7,254 PointsThat line should be made automatically if you choose what sort of layout/the name of the layout when you first make the Activity. If that's what you did, then I'm not sure why it didn't do so. It's of course still good to know what "needs to be done" without always relying on an IDE. After spending an hour on this... I'm sure you'll never forget again. ;)