Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial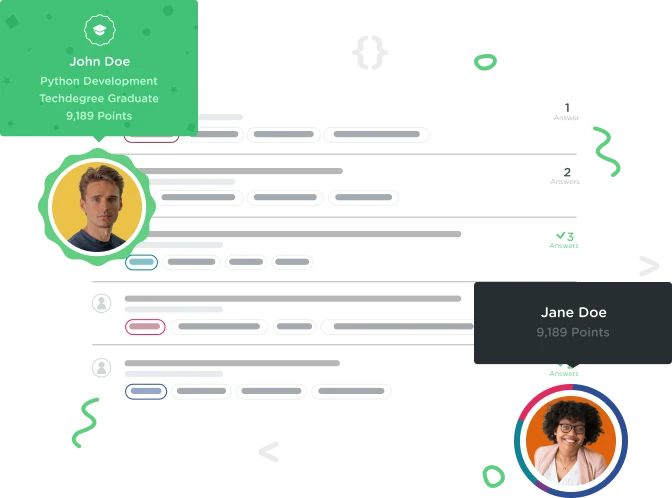

Josh Milbrandt
6,209 PointsApp crashes using view.backgroundColor
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var aButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
aButton.setTitle("Press me!", forState: .Normal)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func buttonPressed() {
view.backgroundColor = UIColor.orangeColor()
}
}
using this code and changing nothing else I get the following error:
2015-05-11 21:52:04.187 Algorythm[7664:278512] -[Algorythm.ViewController buttonPressed:]: unrecognized selector sent to instance 0x7fb69a5b9fd0
2015-05-11 21:52:04.340 Algorythm[7664:278512] *** Terminating app due to uncaught exception 'NSInvalidArgumentException', reason: '-[Algorythm.ViewController buttonPressed:]: unrecognized selector sent to instance 0x7fb69a5b9fd0'
*** First throw call stack:
(
0 CoreFoundation 0x000000010934ec65 __exceptionPreprocess + 165
1 libobjc.A.dylib 0x000000010aeb9bb7 objc_exception_throw + 45
2 CoreFoundation 0x00000001093560ad -[NSObject(NSObject) doesNotRecognizeSelector:] + 205
3 CoreFoundation 0x00000001092ac13c ___forwarding___ + 988
4 CoreFoundation 0x00000001092abcd8 _CF_forwarding_prep_0 + 120
5 UIKit 0x0000000109beeda2 -[UIApplication sendAction:to:from:forEvent:] + 75
6 UIKit 0x0000000109d0054a -[UIControl _sendActionsForEvents:withEvent:] + 467
7 UIKit 0x0000000109cff919 -[UIControl touchesEnded:withEvent:] + 522
8 UIKit 0x0000000109c3b998 -[UIWindow _sendTouchesForEvent:] + 735
9 UIKit 0x0000000109c3c2c2 -[UIWindow sendEvent:] + 682
10 UIKit 0x0000000109c02581 -[UIApplication sendEvent:] + 246
11 UIKit 0x0000000109c0fd1c _UIApplicationHandleEventFromQueueEvent + 18265
12 UIKit 0x0000000109bea5dc _UIApplicationHandleEventQueue + 2066
13 CoreFoundation 0x0000000109282431 __CFRUNLOOP_IS_CALLING_OUT_TO_A_SOURCE0_PERFORM_FUNCTION__ + 17
14 CoreFoundation 0x00000001092782fd __CFRunLoopDoSources0 + 269
15 CoreFoundation 0x0000000109277934 __CFRunLoopRun + 868
16 CoreFoundation 0x0000000109277366 CFRunLoopRunSpecific + 470
17 GraphicsServices 0x000000010d335a3e GSEventRunModal + 161
18 UIKit 0x0000000109bed900 UIApplicationMain + 1282
19 Algorythm 0x0000000109159157 main + 135
20 libdyld.dylib 0x000000010b611145 start + 1
)
libc++abi.dylib: terminating with uncaught exception of type NSException
(lldb)
can anyone help?, the program crashes in main, in the class AppDelegate
2 Answers

Donald White
5,743 PointsI think you're missing self.view in the IBAction? It's trying to reference a selector on an object it's unable to recognize, in this case the view variable, but that doesn't exist as a global.

Josh Milbrandt
6,209 PointsThanks for the quick response, I got the code to work by using buttonPressed(sender: AnyObject)
however when I do things exactly like he does in the video I get the old error, is this maybe a change in Swift 1.2 or something?