Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial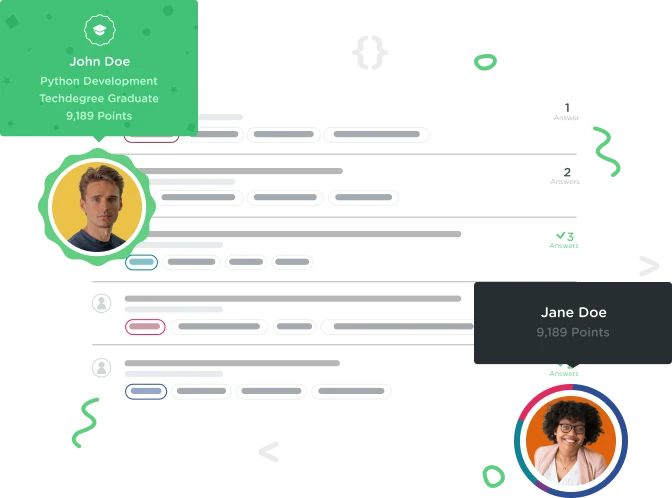
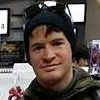
Alexander Eddy
566 PointsApp crashes when I attempt to run it!
I got the following error messages in logcat:
10-06 13:23:09.490 840-840/com.example.alexander.funfacts D/dalvikvm﹕ Not late-enabling CheckJNI (already on) 10-06 13:23:10.210 840-840/com.example.alexander.funfacts D/﹕ HostConnection::get() New Host Connection established 0xb7fa1f00, tid 840 10-06 13:23:10.300 840-840/com.example.alexander.funfacts W/EGL_emulation﹕ eglSurfaceAttrib not implemented 10-06 13:23:10.310 840-840/com.example.alexander.funfacts D/OpenGLRenderer﹕ Enabling debug mode 0 10-06 13:23:11.780 840-840/com.example.alexander.funfacts D/AndroidRuntime﹕ Shutting down VM 10-06 13:23:11.780 840-840/com.example.alexander.funfacts W/dalvikvm﹕ threadid=1: thread exiting with uncaught exception (group=0xb1a56ba8) 10-06 13:23:11.830 840-840/com.example.alexander.funfacts E/AndroidRuntime﹕ FATAL EXCEPTION: main Process: com.example.alexander.funfacts, PID: 840 java.lang.NullPointerException at com.example.alexander.funfacts.FunFactsActivity$1.onClick(FunFactsActivity.java:29) at android.view.View.performClick(View.java:4438) at android.view.View$PerformClick.run(View.java:18422) at android.os.Handler.handleCallback(Handler.java:733) at android.os.Handler.dispatchMessage(Handler.java:95) at android.os.Looper.loop(Looper.java:136) at android.app.ActivityThread.main(ActivityThread.java:5017) at java.lang.reflect.Method.invokeNative(Native Method) at java.lang.reflect.Method.invoke(Method.java:515) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595) at dalvik.system.NativeStart.main(Native Method) 10-06 13:23:14.750 840-840/com.example.alexander.funfacts I/Process﹕ Sending signal. PID: 840 SIG: 9
Thanks for your help!
5 Answers
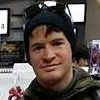
Alexander Eddy
566 PointsHere is the code, showing no errors, but still not working after rebooting the emulator:
package com.example.alexander.funfacts;
import android.app.Activity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends Activity {
private FactBook mFactBook;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
//Declare our View variables and assign them the Views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
final Button showFactButton = (Button) findViewById(R.id.showFactButton);
final View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = mFactBook.getFact();
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
}
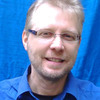
Boris Vukobrat
4,248 PointsRemove final
from View.OnClickListener
statement.
You should also remove it from Button showFactButton
statement. I think this one is set there automatically by Android Studio, and although it will be needed there, you should first learn about it.
You have Null Pointer Exception error at line 29, which means your code puts somewhere a null, where it shouldn't. Since we don't see line numbers, I just can hope removing final
will help : )
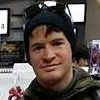
Alexander Eddy
566 PointsHello, I'm still having this issue, even though I deleted final!
Here's the error messages from logcat:
10-08 13:24:39.334 1145-1145/com.example.alexander.funfacts I/Process﹕ Sending signal. PID: 1145 SIG: 9 10-08 13:25:10.044 1198-1198/com.example.alexander.funfacts D/﹕ HostConnection::get() New Host Connection established 0xb769b2d0, tid 1198 10-08 13:25:10.184 1198-1198/com.example.alexander.funfacts W/EGL_emulation﹕ eglSurfaceAttrib not implemented 10-08 13:25:10.214 1198-1198/com.example.alexander.funfacts D/OpenGLRenderer﹕ Enabling debug mode 0 10-08 13:25:12.964 1198-1198/com.example.alexander.funfacts D/AndroidRuntime﹕ Shutting down VM 10-08 13:25:12.964 1198-1198/com.example.alexander.funfacts W/dalvikvm﹕ threadid=1: thread exiting with uncaught exception (group=0xb1accba8) 10-08 13:25:12.984 1198-1198/com.example.alexander.funfacts E/AndroidRuntime﹕ FATAL EXCEPTION: main Process: com.example.alexander.funfacts, PID: 1198 java.lang.NullPointerException at com.example.alexander.funfacts.FunFactsActivity$1.onClick(FunFactsActivity.java:31) at android.view.View.performClick(View.java:4438) at android.view.View$PerformClick.run(View.java:18422) at android.os.Handler.handleCallback(Handler.java:733) at android.os.Handler.dispatchMessage(Handler.java:95) at android.os.Looper.loop(Looper.java:136) at android.app.ActivityThread.main(ActivityThread.java:5017) at java.lang.reflect.Method.invokeNative(Native Method) at java.lang.reflect.Method.invoke(Method.java:515) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595) at dalvik.system.NativeStart.main(Native Method) 10-08 13:25:15.374 1198-1198/com.example.alexander.funfacts I/Process﹕ Sending signal. PID: 1198 SIG: 9
And here's the current code:
package com.example.alexander.funfacts;
import android.app.Activity; import android.graphics.Color; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.RelativeLayout; import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends Activity {
private FactBook mFactBook;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
final RelativeLayout relativeLayout = (RelativeLayout) findViewById(R.id.relativeLayout);
//Declare our View variables and assign them the Views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = mFactBook.getFact();
factLabel.setText(fact);
relativeLayout.setBackgroundColor(Color.RED);
}
};
showFactButton.setOnClickListener(listener);
}
}
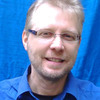
Boris Vukobrat
4,248 PointsWhy don't you just follow the lesson? You've changed the code so now error is at the line 31. Maybe even is not the same one.
You shouldn't go on further if the code doesn't behave as you expect (even with an errors present). Go back to the point where your code worked. Did you turned on line numbers in Android Studio? Why not?
I am guessing you have one of the objects empty (or maybe reference to it), so when your code tries to perform a method from emptiness, returns this error : ).
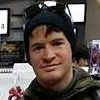
Alexander Eddy
566 PointsI'm fairly certain I followed the lesson, my problem is that I can't identify any differences between my code and Ben's code.
I haven't gone further because my code isn't operating correctly when I try to test it. Yes, I do have line numbers turned on, and there are no error notifications appearing in Android Studio.
An empty object or reference to it is possible, although again, there are no red squigglies in Android Studio.
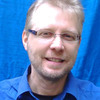
Boris Vukobrat
4,248 PointsOk, so what is in your line 31? Was it the same statement as before at line 29? Perhaps your problem is in FactBook class.
You wouldn't get red error notifications in AS, because syntax is ok.
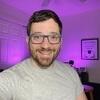
wesley franks
6,198 PointsI am actually experiencing the same issue. My code has been written to match the instructor's.
I can show my code here:
---------------------------FunFactsActivity.java--------------------------------------------------------------
package com.megliosolutions.funfacts;
import android.content.DialogInterface; import android.graphics.Color; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.RelativeLayout; import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends ActionBarActivity {
private FactBook mFactBook;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
//Declare our View variables and assign them the views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
final RelativeLayout relativeLayout = (RelativeLayout) findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String fact = mFactBook.getFact();
// Update the label with our dynamic fact
factLabel.setText("");
relativeLayout.setBackgroundColor(Color.RED);
}
};
showFactButton.setOnClickListener(listener);
}
}
--------------------------------------FactBook.java------------------------------------------------
package com.megliosolutions.funfacts;
import java.util.Random;
/**
-
Created by Wesley on 1/8/2015. */ public class FactBook {
// Member variable (properties about the object) public String[] mFacts = { "Ants stretch when they wake up in the morning.", "Ostriches can run faster than horses.", "Olympic gold medals are actually made mostly of silver.", "You are born with 300 bones; by the time you are an adult you will have 206.", "It takes about 8 minutes for light from the Sun to reach Earth.", "Some bamboo plants can grow almost a meter in just one day.", "The state of Florida is bigger than England.", "Some penguins can leap 2-3 meters out of the water.", "On average, it takes 66 days to form a new habit.", "Mammoths still walked the earth when the Great Pyramid was being built.", "Treehouse is not actually in a tree."}; // Method (abilities: these are things the object can do) public String getFact(){
String fact = ""; // Randomly select a fact Random randomGenerator = new Random(); //Construct a new Random number generator // Assigning an int or number value to choose between int randomNumber = randomGenerator.nextInt(mFacts.length); //fact is being made equal to randomNumber adding an empty fact = randomNumber + ""; fact = mFacts[randomNumber]; return fact;
}
}
I am not sure where my issue is. with the Null pointer error.
Kate Hoferkamp
5,205 PointsKate Hoferkamp
5,205 PointsCan you post your code?
Edit: Try and close and reopen your emulator, then launch the app. That may fix it.