Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial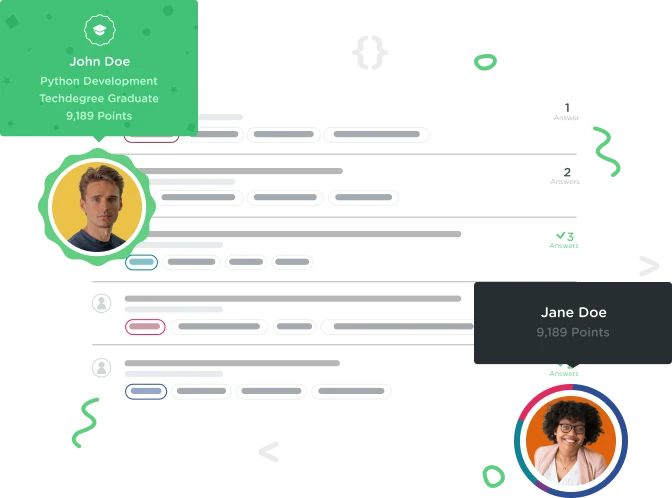

welsen ho
1,330 PointsApp crashes when loading the first page
here is my code pls help
package com.example.welse.interactivestory.ui;
import android.content.Intent;
import android.graphics.drawable.Drawable;
import android.support.v4.content.ContextCompat;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import com.example.welse.interactivestory.R;
import com.example.welse.interactivestory.Story;
import com.example.welse.interactivestory.model.page;
public class StoryActivity extends AppCompatActivity {
public static final String TAG = StoryActivity.class.getSimpleName();
private Story story;
private ImageView storyImageView;
private TextView textView;
private Button choice1Button;
private Button choice2Button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
storyImageView = findViewById(R.id.storyImageView);
textView = findViewById(R.id.textView);
choice1Button = findViewById(R.id.choice1Button);
choice2Button = findViewById(R.id.choice2Button);
Intent intent = getIntent();
String name = intent.getStringExtra("name");
if(name == null || name.isEmpty()){
name = "friend";
}
Log.d(TAG, name );
story = new Story();
loadPage(0);
}
private void loadPage(int pageNumber) {
page index = story.getPage(pageNumber);
Drawable image = ContextCompat.getDrawable(this, index.getImageId());
storyImageView.setImageDrawable(image);
}
}
package com.example.welse.interactivestory;
import com.example.welse.interactivestory.model.choice;
import com.example.welse.interactivestory.model.page;
/**
* Created by welse on 2018/2/17.
*/
public class Story {
private page[] Pages;
public Story(){
Pages[0] = new page(R.drawable.page0, R.string.page0
,new choice(R.string.page0_choice1,1)
,new choice(R.string.page0_choice2,2));
Pages[1] = new page(R.drawable.page1,
R.string.page1,
new choice(R.string.page1_choice1, 3),
new choice(R.string.page1_choice2, 4));
Pages[2] = new page(R.drawable.page2,
R.string.page2,
new choice(R.string.page2_choice1, 4),
new choice(R.string.page2_choice2, 6));
Pages[3] = new page(R.drawable.page3,
R.string.page3,
new choice(R.string.page3_choice1, 4),
new choice(R.string.page3_choice2, 5));
Pages[4] = new page(R.drawable.page4,
R.string.page4,
new choice(R.string.page4_choice1, 5),
new choice(R.string.page4_choice2, 6));
Pages[5] = new page(R.drawable.page5, R.string.page5);
Pages[6] = new page(R.drawable.page6, R.string.page6);
}
public page getPage(int pageNumber) {
if (pageNumber >= Pages.length){
pageNumber = 0;
}
return Pages[pageNumber];
}
}
package com.example.welse.interactivestory.model;
/**
* Created by welse on 2018/2/15.
*/
public class page {
private int imageId;
private int textId;
private choice choice1;
private choice choice2;
private boolean isFinalPage = false;
public page(int imageId, int textId) {
this.imageId = imageId;
this.textId = textId;
this.isFinalPage = true;
}
public page(int imageId, int textId, choice choice1, choice choice2) {
this.imageId = imageId;
this.textId = textId;
this.choice1 = choice1;
this.choice2 = choice2;
}
public boolean isFinalPage() {
return isFinalPage;
}
public void setFinalPage(boolean finalPage) {
isFinalPage = finalPage;
}
public int getImageId() {
return imageId;
}
public void setImageId(int imageId) {
this.imageId = imageId;
}
public int getTextId() {
return textId;
}
public void setTextId(int textId) {
this.textId = textId;
}
public choice getChoice1() {
return choice1;
}
public void setChoice1(choice choice1) {
this.choice1 = choice1;
}
public choice getChoice2() {
return choice2;
}
public void setChoice2(choice choice2) {
this.choice2 = choice2;
}
}

welsen ho
1,330 Pointsthis is what it show when it crashed
--------- beginning of crash
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.welse.interactivestory, PID: 3653
java.lang.RuntimeException: Unable to start activity
ComponentInfo{com.example.welse.interactivestory/com.example.welse.interactivestory.ui.StoryActivity}:
java.lang.NullPointerException: Attempt to write to null array
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2665)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2726)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1477)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6119)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:886)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:776)
Caused by: java.lang.NullPointerException: Attempt to write to null array
at com.example.welse.interactivestory.Story.<init>(Story.java:15)
at com.example.welse.interactivestory.ui.StoryActivity.onCreate(StoryActivity.java:47)
at android.app.Activity.performCreate(Activity.java:6679)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1118)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2618)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2726)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1477)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6119)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:886)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:776)
Application terminated.
1 Answer
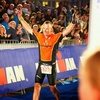
Steve Hunter
57,712 PointsHi there,
You have a NullPointerException
because the array is null
. The relevant part of your error is: Caused by: java.lang.NullPointerException: Attempt to write to null array at com.example.welse.interactivestory.Story.<init>(Story.java:15)
.
This is happening because you haven't created your array properly. Have a look at this video at 1m05s. You need to create a new
array of Page
. You've missed that line of code within onCreate
so you array hasn't been created properly.
As a side note. You have called your class page
(lowercase) and your array Pages
(uppercase). I'd recommend refactoring that so that your class is uppercase and your instance is lowercase. That's the standard notation. Not adhering to it will cause confusion later in the course.
Steve.

welsen ho
1,330 PointsThank you very much!!! My problem solved Btw how do you know there is an array problem in my code ?
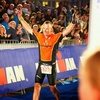
Steve Hunter
57,712 PointsThe error messages from the Logcat show that there was an attempt to write to a null array.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi there,
It would really help if you could post the error and a copy of the Logcat output when the app crashes.
Steve.