Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial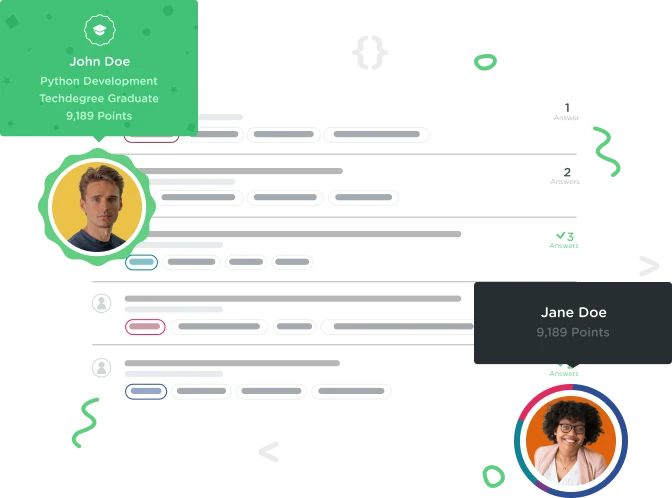
adnanalvee2
6,862 PointsApp doesn't go back to Inbox view automatically after Sending any "Picture/Video"
My app successfully sends a picture/video and outputs the "Success" message but still stays in the friend selection screen.
Here is my code.
How do I move it to Inbox immediately after pressing SEND just like Ben?
package com.dipnsa.dipdip;
import java.util.ArrayList;
import java.util.List;
import android.app.AlertDialog;
import android.app.ListActivity;
import android.net.Uri;
import android.os.Bundle;
import android.support.v4.app.NavUtils;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.view.Window;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.Toast;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseFile;
import com.parse.ParseObject;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import com.parse.SaveCallback;
public class RecipentsActivity extends ListActivity {
public static final String TAG = RecipentsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
protected Uri mMediaUri;
protected String mFileType;
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_recipents, container,
false);
return rootView;
}
public void onResume() {
super.onResume();
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
setProgressBarVisibility(true);
ParseQuery<ParseUser> query = mFriendsRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
mFriendsRelation.getQuery().findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
setProgressBarVisibility(false);
mMediaUri = getIntent().getData();
mFileType = getIntent().getExtras().getString(ParseConstants.KEY_FILE_TYPE);
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for(ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
getListView().getContext(),
android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
}
else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(RecipentsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.recipents, menu);
mSendMenuItem = menu.getItem(0);
return true;
};
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
switch (item.getItemId()) {
case android.R.id.home:
NavUtils.navigateUpFromSameTask(this);
return true;
case R.id.action_send:
ParseObject message = createMessage();
if (message == null) {
//error
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage(R.string.error_select_error)
.setTitle("Test Title")
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
} else {
send(message);
}
//send(message);
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
if (l.getCheckedItemCount() >0 ) {
mSendMenuItem.setVisible(true);
}
else {
mSendMenuItem.setVisible(false);
}
}
protected ParseObject createMessage() {
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
message.put(ParseConstants.KEY_RECIPENT_IDS, getRecipentIds());
message.put(ParseConstants.KEY_FILE_TYPE, mFileType);
byte[] fileBytes = FileHelper.getByteArrayFromFile(this, mMediaUri);
if (fileBytes == null) {
return null;
}
else {
if (mFileType.equals(ParseConstants.TYPE_IMAGE)) {
fileBytes = FileHelper.reduceImageForUpload(fileBytes);
}
String fileName = FileHelper.getFileName(this, mMediaUri, mFileType);
ParseFile file = new ParseFile (fileName, fileBytes);
message.put(ParseConstants.KEY_FILE, file);
return message;
}
}
protected ArrayList<String> getRecipentIds() {
ArrayList<String> recipentIds = new ArrayList<String>();
for (int i = 0; i<getListView().getCount(); i++) {
if (getListView().isItemChecked(i)) {
recipentIds.add(mFriends.get(i).getObjectId());
}
}
return recipentIds;
}
protected void send(ParseObject message) {
message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e == null) {
Toast.makeText(RecipentsActivity.this, R.string.message_sent, Toast.LENGTH_LONG).show();
}
else {
AlertDialog.Builder builder = new AlertDialog.Builder(RecipentsActivity.this);
builder.setMessage(R.string.error_sending_message)
.setTitle("Error")
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}