Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial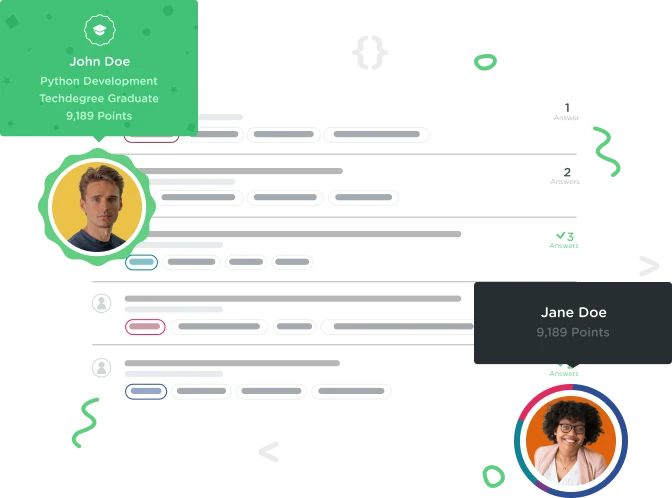
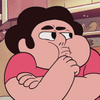
Ludwing Najera
4,596 PointsApp "fatal error" when clicking "show another fun fact" button. It's supposed to change background color!
My FunFact app crashes with this message in the console "fatal error: Array index out of range (lldb)"
It has a breakpoint on these two lines.
view.backgroundColor = colorWheel.randomColor()
And
return factsArray[randomNumber]
10 Answers

Stone Preston
42,016 Pointsyour var randomNumber needs to be Int(unsignedRandomNumber) not Int(unsignedColorsArray):
func randomColor() -> UIColor {
var unsignedColorsArray = UInt32(colorsArray.count)
var unsignedRandomNumber = arc4random_uniform (unsignedColorsArray)
// cast the unsignedRandomNumber to an Int, not unsignedColorsArray
var randomNumber = Int(unsignedRandomNumber)
return colorsArray[randomNumber]
}

Stone Preston
42,016 Pointspost the randomColor method thats implemented in the colorWheel class
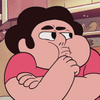
Ludwing Najera
4,596 Pointshere
func randomColor() -> UIColor {
var unsignedColorsArray = UInt32(colorsArray.count)
var unsignedRandomNumber = arc4random_uniform (unsignedColorsArray)
var randomNumber = Int(unsignedColorsArray)
return colorsArray[randomNumber]
}
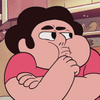
Ludwing Najera
4,596 Pointswhen i do that, i get another error saying "Expected declaration"

Stone Preston
42,016 Pointsit could be the fact you have a space after the arc4random_uniform method name, try removing that and making sure you spelled everything correctly
func randomColor() -> UIColor {
var unsignedColorsArray = UInt32(colorsArray.count)
// remove the space
var unsignedRandomNumber = arc4random_uniform(unsignedColorsArray)
// cast the unsignedRandomNumber to an Int, not unsignedColorsArray
var randomNumber = Int(unsignedRandomNumber)
return colorsArray[randomNumber]
}
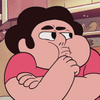
Ludwing Najera
4,596 Pointsi removed the space and the error still lingers

Stone Preston
42,016 Pointspost your full code for the struct
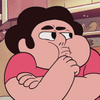
Ludwing Najera
4,596 Pointsstruct ColorWheel {
let colorsArray = [
UIColor(red: 90/255.0, green: 187/255.0, blue: 181/255.0, alpha: 1.0), //teal color
UIColor(red: 222/255.0, green: 171/255.0, blue: 66/255.0, alpha: 1.0), //yellow color
UIColor(red: 223/255.0, green: 86/255.0, blue: 94/255.0, alpha: 1.0), //red color
UIColor(red: 239/255.0, green: 130/255.0, blue: 100/255.0, alpha: 1.0), //orange color
UIColor(red: 77/255.0, green: 75/255.0, blue: 82/255.0, alpha: 1.0), //dark color
UIColor(red: 105/255.0, green: 94/255.0, blue: 133/255.0, alpha: 1.0), //purple color
UIColor(red: 85/255.0, green: 176/255.0, blue: 112/255.0, alpha: 1.0), //green color
]
func randomColor() -> UIColor {
var unsignedColorsArray = UInt32(colorsArray.count)
var unsignedRandomNumber = arc4random_uniform(unsignedColorsArray)
var randomNumber = Int(unsignedRandomNumber)
return colorsArray[randomNumber]
}
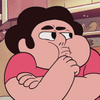
Ludwing Najera
4,596 Pointsokay i fixed it so i never closed the struct brackets and thats what was causing the problems

Stone Preston
42,016 Pointsyou are missing the closing brace of your function:
struct ColorWheel {
let colorsArray = [
UIColor(red: 90/255.0, green: 187/255.0, blue: 181/255.0, alpha: 1.0), //teal color
UIColor(red: 222/255.0, green: 171/255.0, blue: 66/255.0, alpha: 1.0), //yellow color
UIColor(red: 223/255.0, green: 86/255.0, blue: 94/255.0, alpha: 1.0), //red color
UIColor(red: 239/255.0, green: 130/255.0, blue: 100/255.0, alpha: 1.0), //orange color
UIColor(red: 77/255.0, green: 75/255.0, blue: 82/255.0, alpha: 1.0), //dark color
UIColor(red: 105/255.0, green: 94/255.0, blue: 133/255.0, alpha: 1.0), //purple color
UIColor(red: 85/255.0, green: 176/255.0, blue: 112/255.0, alpha: 1.0), //green color
]
func randomColor() -> UIColor {
var unsignedColorsArray = UInt32(colorsArray.count)
var unsignedRandomNumber = arc4random_uniform(unsignedColorsArray)
var randomNumber = Int(unsignedRandomNumber)
return colorsArray[randomNumber]
// add the closing brace
}
}
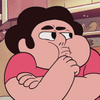
Ludwing Najera
4,596 Pointsyeah i fixed that and it worked