Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial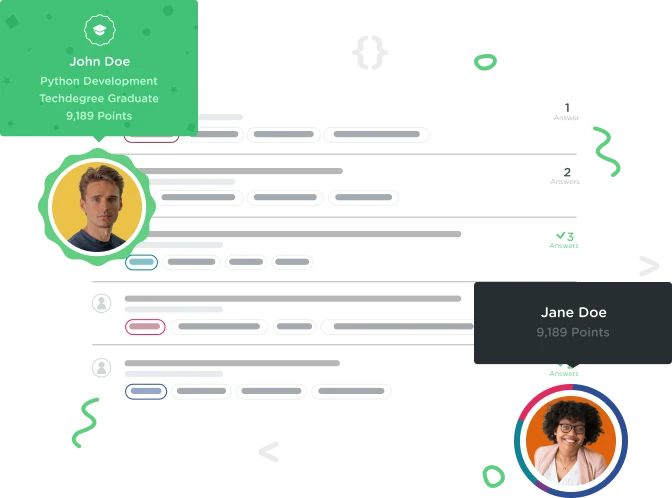

Branden Bird
2,481 PointsApp keeps crashing.
I can't keep it from crashing, I don't have any errors but it still happens.
Here is the LogCat:
03-20 01:53:15.393: D/AndroidRuntime(1317): Shutting down VM
03-20 01:53:15.393: W/dalvikvm(1317): threadid=1: thread exiting with uncaught exception (group=0xb2a77ba8)
03-20 01:53:15.403: E/AndroidRuntime(1317): FATAL EXCEPTION: main
03-20 01:53:15.403: E/AndroidRuntime(1317): Process: com.example.crystalball, PID: 131703-20 01:53:15.403: E/AndroidRuntime(1317): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.crystalball/com.example.crystalball.MainActivity}: java.lang.NullPointerException
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2195)
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2245)
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.app.ActivityThread.access$800(ActivityThread.java:135)
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1196)
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.os.Handler.dispatchMessage(Handler.java:102)
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.os.Looper.loop(Looper.java:136)
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.app.ActivityThread.main(ActivityThread.java:5017)
03-20 01:53:15.403: E/AndroidRuntime(1317): at java.lang.reflect.Method.invokeNative(Native Method)
03-20 01:53:15.403: E/AndroidRuntime(1317): at java.lang.reflect.Method.invoke(Method.java:515)
03-20 01:53:15.403: E/AndroidRuntime(1317): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779)
03-20 01:53:15.403: E/AndroidRuntime(1317): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595)
03-20 01:53:15.403: E/AndroidRuntime(1317): at dalvik.system.NativeStart.main(Native Method)
03-20 01:53:15.403: E/AndroidRuntime(1317): Caused by: java.lang.NullPointerException
03-20 01:53:15.403: E/AndroidRuntime(1317): at com.example.crystalball.MainActivity.onCreate(MainActivity.java:26)
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.app.Activity.performCreate(Activity.java:5231)
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1087)
03-20 01:53:15.403: E/AndroidRuntime(1317): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2159)
03-20 01:53:15.403: E/AndroidRuntime(1317): ... 11 more
03-20 01:53:18.553: I/Process(1317): Sending signal. PID: 1317 SIG: 9

Branden Bird
2,481 Points Here is my MainActivity.java file:
package com.example.crystalball;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v7.app.ActionBarActivity;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declare our View variables
final TextView answerLabel = (TextView) findViewById(R.id.textView1);
Button getAnswerButton = (Button) findViewById(R.id.button1);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// The button was clicked, so update the answer label with an answer
String answer = "Yes";
answerLabel.setText(answer);
}
});
if (savedInstanceState == null) {
getSupportFragmentManager().beginTransaction()
.add(R.id.container, new PlaceholderFragment())
.commit();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
/**
* A placeholder fragment containing a simple view.
*/
public static class PlaceholderFragment extends Fragment {
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_main, container, false);
return rootView;
}
}
}

Branden Bird
2,481 Points When going through the debugger line by line it fails in the ThreadGroup.class
/**
* Handles uncaught exceptions. Any uncaught exception in any {@code Thread}
* is forwarded to the thread's {@code ThreadGroup} by invoking this
* method.
*
* <p>New code should use {@link Thread#setUncaughtExceptionHandler} instead of thread groups.
*
* @param t the Thread that terminated with an uncaught exception
* @param e the uncaught exception itself
*/
public void uncaughtException(Thread t, Throwable e) {
if (parent != null) {
parent.uncaughtException(t, e); <--- This is the line it fails at
} else if (Thread.getDefaultUncaughtExceptionHandler() != null) {
// TODO The spec is unclear regarding this. What do we do?
Thread.getDefaultUncaughtExceptionHandler().uncaughtException(t, e);
} else if (!(e instanceof ThreadDeath)) {
// No parent group, has to be 'system' Thread Group
e.printStackTrace(System.err);
}
}
/**
* Called by the Thread constructor.
*/
4 Answers

Branden Bird
2,481 PointsI feel like an idiot, I didn't follow instructions after creating the new project and delete the fragment related code.
Under teachers notes the fourth bullet down is what needs to be done.
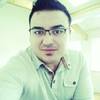
. Ali
9,799 PointsLooks like you having a problem with your main activity.. which is com.example.crystalball.MainActivity..
check if you have any problems outlined to u by eclipse

Branden Bird
2,481 PointsThere were no errors with the red exclamation in the mainactivity.java file, I can't post my code at this time since I am not near my workstation right now.
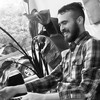
Sage Elliott
30,003 PointsDid you figure this out? Mine crashes immediately.

Branden Bird
2,481 PointsNo I did not, I tried searching and could not turn up anything. I just moved back to the web developers track. But will keep looking for a solution.
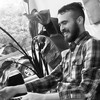
Sage Elliott
30,003 PointsSame here. If I figure it out first ill let you know.
Branden Bird
2,481 PointsBranden Bird
2,481 PointsMy MainActivity.java generates a lot more code than what is in the video.