Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial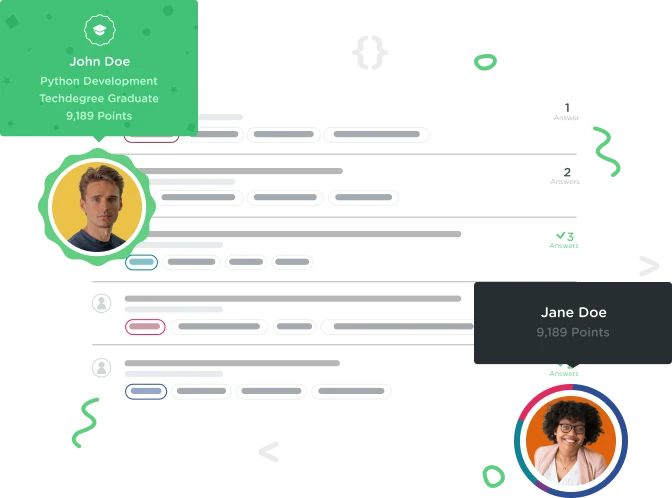

Robbin Kalaamalho
1,571 PointsApp keeps crashing
Whenever I start my Emulator my app crashes, any suggestions?
public class FunFactsActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
final String[] facts= {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built." };
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our View variables and assign the the Views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
// The button was clicked so update the fact label with a new fact
String fact = "";
//Randomly select a fact.
Random randomGenerator = new Random(); //construct a new number generator
int randomNumber = randomGenerator.nextInt(fact.length());
fact = facts[randomNumber];
//Update the label with our dynamic fact.
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.fun_facts, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
2 Answers
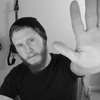
Jeremiah Shore
31,168 Pointslooks like the following line
int randomNumber = randomGenerator.nextInt(fact.length());
needs to be (facts instead of fact and no () )
int randomNumber = randomGenerator.nextInt(facts.length);
If you have any further issues be sure to post them. Also the stack trace from the log is useful in helping you find where the errors are. If you don't understand the error message, google is your friend.

Robbin Kalaamalho
1,571 PointsOh Thanks it automatically added () to the code when I typed fact.length Thanks for the info ill make the changes as soon as I get home and ill let you know how it goes :)
Robbin Kalaamalho
1,571 PointsRobbin Kalaamalho
1,571 Pointswhen I correct the issue to
int randomNumber = randomGenerator.nextInt(facts.length);
the word Length turns Red and when I try to run the program it gives the following errors
Error:(44, 64) error: cannot find symbol variable length
Error:Execution failed for task ':app:compileDebugJava'.
Information:BUILD FAILED
what should I do?
Jeremiah Shore
31,168 PointsJeremiah Shore
31,168 PointsYou say "facts.length" but also "Length ", you are using the lower case version right? I copied your code and mine is compiling fine. Let me know and I can try to take a closer look.