Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial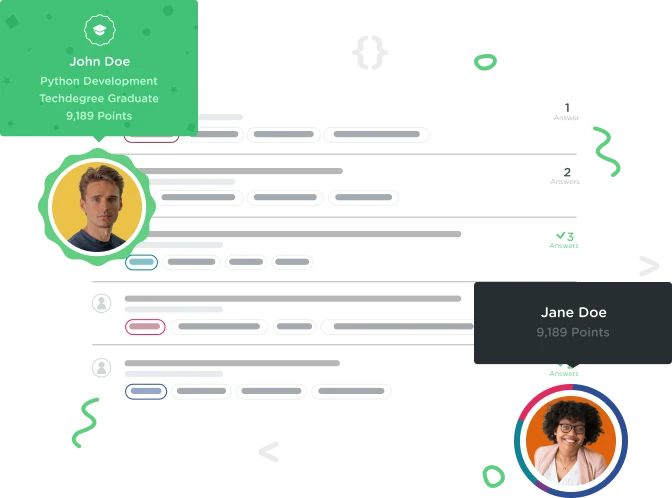

Victoria M
8,730 PointsApp not working when I try to load it
When I try to load the random number changes my app won't load and I am prompted with an error to change var to let. Does any one know why? This is what I have in factbook.swift:
import Foundation
struct FactBook { let factsArray = [ "Ants stretch when they wake up in the morning.", "Ostriches can run faster than horses.", "Olympic gold medals are actually made mostly of silver.", "You are born with 300 bones; by the time you are an adult you will have 206.", "It takes about 8 minutes for light from the Sun to reach Earth.", "Some bamboo plants can grow almost a meter in just one day.", "The state of Florida is bigger than England.", "Some penguins can leap 2-3 meters out of the water.", "On average, it takes 66 days to form a new habit.", "Mammoths still walked the earth when the Great Pyramid was being built." ]
func randomFact() -> String {
var unsignedArrayCount = UInt32(factsArray.count)
var unsignedRandomNumber = arc4random_uniform(unsignedArrayCount)
var randomNumber = Int(unsignedRandomNumber)
return factsArray[randomNumber]
}
}
This is what I have in my view controller:
import UIKit
class ViewController: UIViewController { @IBOutlet weak var funFactLabel: UILabel!
let factBook = FactBook()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
funFactLabel.text = factBook.randomFact()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func showFunFact() {
funFactLabel.text = factBook.randomFact()
}
}
1 Answer
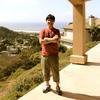
Richard Lu
20,185 PointsSo from what I understand, your question is why XCode is prompting you to change your var to a let. The reason comes directly from Apples documentation
"If a stored value in your code is not going to change, always declare it as a constant with the let keyword. Use variables only for storing values that need to be able to change."
Source: http://tinyurl.com/mlw75pm
In your case factBook never changes.
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var funFactLabel: UILabel!
let factBook = FactBook() // THIS NEVER CHANGES
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
funFactLabel.text = factBook.randomFact() // IT IS ONLY UTILIZED
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func showFunFact() {
funFactLabel.text = factBook.randomFact()
}
}
I hope I've helped :)