Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial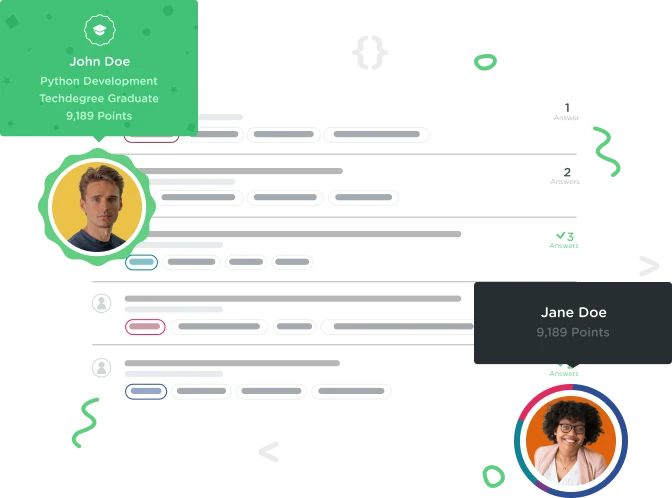

David Brown
2,619 Points"Append"
I've gotten to this point, and I get the Switch statements method fine, but for some reason the Append thing is still tripping me up. In this situation, we've got countries and capitals, so we switch key { case "BEL": europeanCapitals.append(value) } to make it work, but even though it works I'm still not clear on how or why... weird, I know. Can anyone please break down the whole Appending thing here? Thanks!
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
// End code
}
1 Answer
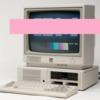
kols
27,007 PointsHi, David — I'll go ahead and say up-front that this should make a lot more sense once you get to functions/methods (if you haven't already); but hopefully this will help in the meantime...
For this challenge, the instructor has gone ahead and created empty arrays for you up at the top that he wants you to programmatically populate, and provided you with a dictionary ('world') containing the information he wants you to add. Here they are again for reference:
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
Specifically, he asks that you fill-in these empty arrays by iterating over the dictionary using a for-in statement (which he provides) and using a switch statement, which you said you understand, so I'll continue on. (More on switch statements here if anyone else is curious.)
Here's the code challenge answer using a switch statement, in which we switch on the dictionary's keys:
for (key, value) in world {
switch key {
case "BEL", "LIE", "BGR":
europeanCapitals.append(value)
case "IND", "VNM":
asianCapitals.append(value)
default: otherCapitals.append(value)
}
}
All good to this point? Cool, moving on...
Adding to Arrays using the Append Method
For our purposes, the two main ways that you can add an item to arrays is by:
- Using the append method — generally seems preferred, and is what the code challenge asks for in this example
- Using subscript syntax — I won't go into adding to arrays using subscript syntax here since they ask you to use the other option, but you can read more about it if you'd like in Swift Documentation | Collection Types under the heading "Accessing and Modifying an Array"
OK — Here's a single line again from the above code:
europeanCapitals.append(value)
We're appending to each of the arrays by first typing out the array name:
europeanCapitals
Then, we call the append method on it (which is simply a pre-defined method for adding items to an array, and is the same as calling any other function/method on an instance).
Note: If you haven't gotten to functions/methods yet, this should make a lot more sense later; don't worry about it if you haven't, though, just know that to add an item to an array, you can always just use the append method as shown below, using a . then append and then () :
.append()
Then, within the parenthesis, you type out whatever you want to append to the array:
europeanCapitals.append(value)
We type value here because - in this specific example - we are iterating over a dictionary's key-value pairs to populate these arrays. Meaning, whenever it finds a specific key (e.g, "BEL", "LIE", "BGR") in the dictionary, we've told it to append the corresponding value ("Brussels", "Vaduz", "Sofia") to this specific array.
Here are some more examples not using the code challenge to illustrate this further:
// To add a new name ('Joe') to an array ('myFriends'):
myFriends.append("Joe")
// To add multiple items to an array ('toDoList')
toDoList.append("Buy Groceries", "Send mom email", "Watch Season 2 of Stranger Things")
I hope that helps!!
You may want to go back and quickly review the videos for Collections (specifically Arrays and Dictionaries); I know that it always helps me to go back and review concepts that I'm a little fuzzy on (watch on double speed and you can go through them in just a few minutes).
Also, it would also probably be a good idea to check out the Swift Documentation on Collection Types to make sure you're solid on those foundational concepts before moving forward.