Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial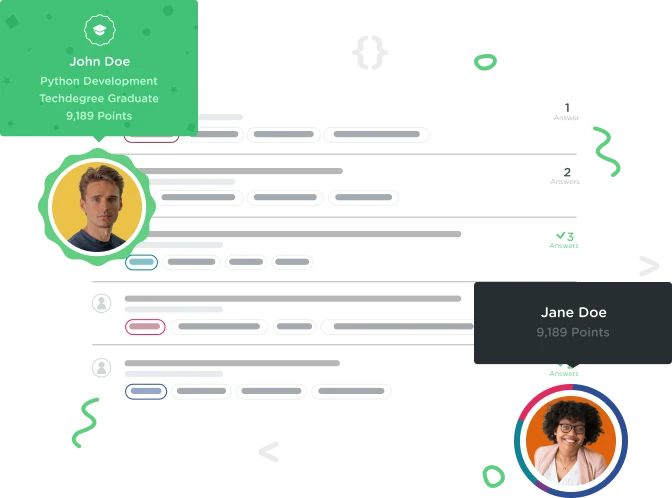

Tanner B
926 Points.append() or while loop problem?
So, when I run the program, regardless of how many names I enter, the roster prints, but each player's name is that of the last name entered. (ex: four players entered, last player entered is Josh, it prints: #1 Josh, #2 Josh, #3 Josh, #4 Josh). What's causing the first few names to be replaced with the last?
player_list = []
new_player = input("Would you like to add a player to the list? (Yes/No) ") while new_player.lower() == 'yes': player_name = input("Please enter their name: ") player_list.append(player_name) new_player = input("Would you like to add another player? (Yes/No) ")
roster_size = len(player_list) if roster_size == 1: print("The current roster has {} player.".format(roster_size)) else: print("The current roster has {} players.".format(roster_size))
player_number = 1 for player in player_list: print("#{} {}".format(player_number, player_name)) player_number += 1
goalie = input("Please select a goalie by selecting the player number. (1-{})".format(len(player_list)))
goalie = int(goalie) print("Great! The goalie for the game will be {}.".format(players[goalie - 1]))
3 Answers

KRIS NIKOLAISEN
54,971 PointsThe names are all the same because you are using variable player_name
. This has the value of the last assignment which would be the last name received from the user as input. Instead the code should be:
for player in player_list:
print("#{} {}".format(player_number, player))
player_number += 1
You also have an error here:
print("Great! The goalie for the game will be {}.".format(players[goalie - 1]))
players
is undefined. I think you meant to use player_list
:
print("Great! The goalie for the game will be {}.".format(player_list[goalie - 1]))

jvar
3,251 PointsYou should have two separate variables: one to store each player added, which you will append to the players' list, and the other to store the answer to yes or no. Here is the portion of my practice looks like:
# TODO Create an empty list to maintain the player names
players =[]
# TODO Ask the user if they'd like to add players to the list.
add_player = input("Would your like to add a player to the list? ")
# If the user answers "Yes", let them type in a name and add it to the list.
while add_player == "y":
player = input("Please enter a name for the player you'd like to add: ")
players.append(player)
add_player = input("Would your like to add a player to the list? ")
# If the user answers "No", print out the team 'roster'
print(players)
```

Saikat Chowdhury
3,128 PointsFull code is working fine only 2 places you misses variable name .
1st : I have updated, within for loop 2nd argument of .format
print("#{} {}".format(player_number, player))
2nd : I have updated the list name to "player_list"
print("Great! The goalie for the game will be {}.".format(player_list[goalie - 1]))