Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial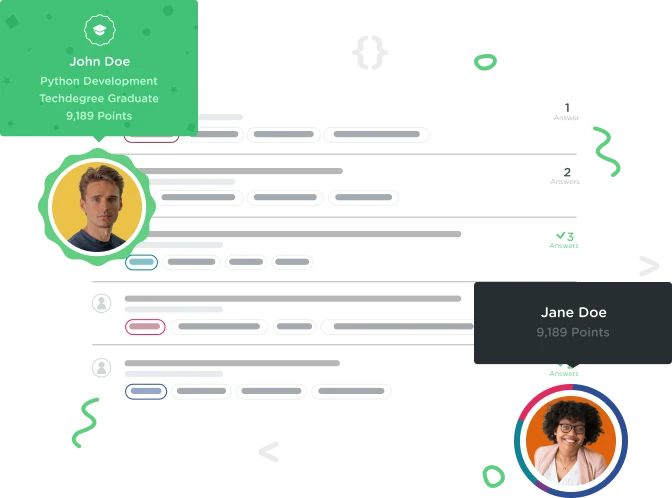

Steven Rayzman
2,423 PointsAppending Button To List Item
I am creating an unordered list from an array. However I would like the list items to be buttons who's text are from the array? How would I accomplish this?
2 Answers

LaVaughn Haynes
12,397 PointsIf you were looking for plain JavaScript then this would work
<body>
<div id="output"></div>
<script>
var theList = ['Update', 'Cancel', 'Delete'];
var output = document.getElementById('output');
var getButtonList = function(items){
var html = '<ul>';
for(var item in items){
html += '<li><button>' + items[item] + '</button></li>';
}
html += '</ul>';
return html;
};
output.innerHTML = getButtonList( theList );
</script>
</body>
That's going to output this:
<div id="output">
<ul>
<li><button>Update</button></li>
<li><button>Cancel</button></li>
<li><button>Delete</button></li>
</ul>
</div>
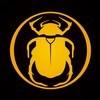
rydavim
18,814 PointsTo get a solid solution for your specific problem, you'll probably need to supply your code or some more information about your goal.
Here is a quick and dirty solution to my understanding of your problem, using JQuery.
// Things I am assuming:
// - You have an unordered list on your html page with the id "buttonList".
// - You have an array of strings (myArray below).
// Example Array
var myArray = ["Alpha", "Bravo", "Charlie", "Delta", "Echo", "Foxtrot"];
// Cycle over the array.
for (var i = 0; i < myArray.length; i++) {
// Create a list item.
var $exampleItem = $("<li></li>");
// Create a button.
var $exampleButton = $("<button></button>");
// Set the button's text to be the current array item.
$exampleButton.text(myArray[i]);
// Append the button to the list item.
var $newButton = $exampleItem.append($exampleButton);
// Append this to the unordered list.
$("#buttonList").append($newButton);
}
// There is probably a better way to do this, but this should work.