Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial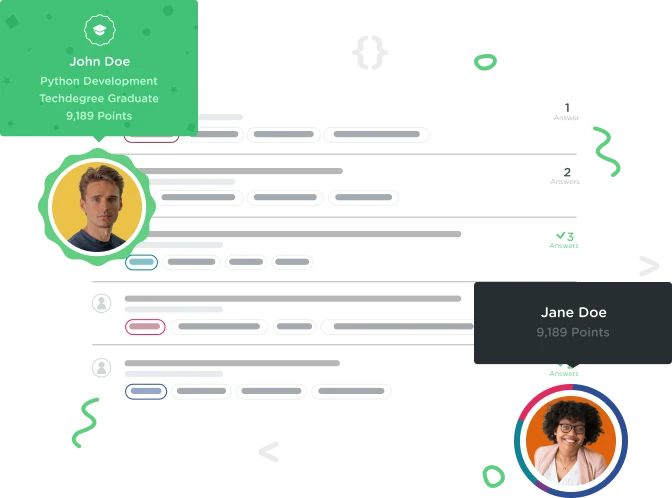

Kevin Dunn
6,623 PointsAppending child elements not working
Hi, I have a problem with appending the li elements to the ul. I keep getting this error in the console: "Uncaught TypeError: Cannot read property 'addEventListener' of null". Hope someone could help me out. Thanks
Here is my code:
const toggleList = document.getElementById('toggleList');
const list = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const addItemInput = document.querySelector('addItemInput');
const addItemButton = document.querySelector('addItemButton');
//const ul = document.querySelector('ul');
toggleList.addEventListener('click', function(){
if(list.style.display == 'none'){
toggleList.textContent = 'Hide List';
list.style.display = 'block';
}
else{
toggleList.textContent = 'Show List';
list.style.display = "none";
}
});
descriptionButton.addEventListener('click', ()=> {
descriptionP.innerHTML = descriptionInput.value + ': ';
});
addItemButton.addEventListener('click', ()=>{
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = addItemInput.value;
ul.appendChild('li');
});
4 Answers

Kevin Dunn
6,623 PointsThanks for the replying guys. Actually I figured out the problem. I used quotes when trying to append the li element to the ul. So I had to remove the quotes.
ul.appendChild(li);
Also when using the document.querySelector, I had to make the search more specific in the parameters like so:
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
But why is it that the querySelector cant find the element if I just enter the name of the class without the the element tags 'input' and 'button'?

ve
5,048 PointsThis error message normally means that your constant "toggleList" is empty, are you sure that your Element has the ID "toggleList"?
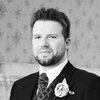
Joel Bardsley
31,249 PointsThere's a problem with your querySelector selectors for addItemInput and addItemButton - you need to include a # or a . for id/class selectors respectively.
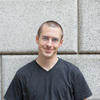
Nathan Brenner
35,844 PointsIn this case, you need the element name and the class name to be specific enough to get the intended node.
For future debugging, when you get that error message, add console.log(node)
where node
is the dom node that you have the event listening on.
nathanielcusano
9,808 Pointsnathanielcusano
9,808 PointsIt's not working because it's supposed to be