Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial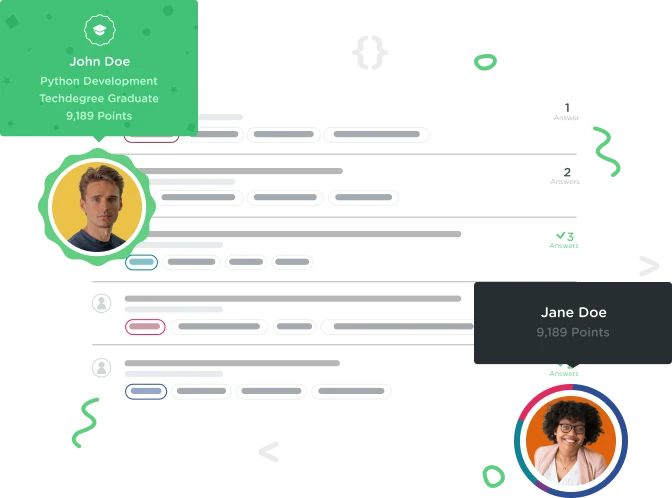
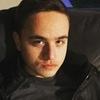
Emre Karakuz
9,162 PointsAppending Nodes in JavaScript
Hey All,
This is my code which is being taught about appending nodes in this video : https://teamtreehouse.com/library/appending-nodes
this code is about creating new DOM elements on page and appending nodes. You'll see what it is doing when you run the code.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<button id="toggleList">Hide List</button>
<div class="list">
<p class="description">Things that are in list</p>
<input type="text" class="description">
<button class="description">Change List Description</button>
<ul>
<li>grapes</li>
<li>amethyst</li>
<li>lavender</li>
<li>plums</li>
</ul>
<input type="text" class="addItemInput">
<button class="addItemButton">Add Item</button>
</div>
<script src="app.js"></script>
</body>
</html>
const toggleList = document.getElementById('toggleList');
const listDiv = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
toggleList.addEventListener('click', () => {
if(listDiv.style.display == 'none') {
toggleList.textContent = 'Hide List';
listDiv.style.display = 'block';
} else {
toggleList.textContent = 'Show List';
listDiv.style.display = 'none';
}
});
descriptionButton.addEventListener('click' , () => {
descriptionP.innerHTML=descriptionInput.value + ':';
descriptionInput.value = '';
});
addItemButton.addEventListener('click' , () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = addItemInput.value;
ul.appendChild(li);
addItemInput.value = '';
});
this code works well, but I got a question. This code adds <li> elements under <ul> and this process is being done with this code :
addItemButton.addEventListener('click' , () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = addItemInput.value;
ul.appendChild(li);
addItemInput.value = '';
});
other parts are not about adding an element except variables. but when I write something wrong in other parts of code like :
button.addEventListener('click' , () => {
descriptionP.innerHTML=descriptionInput.value + ':';
descriptionInput.value = '';
});
instead
descriptionButton.addEventListener('click' , () => {
descriptionP.innerHTML=descriptionInput.value + ':';
descriptionInput.value = '';
});
Browser cannot add an item to the list but they do different things
same for writing :
List.addEventListener('click', () => {
if(listDiv.style.display == 'none') {
toggleList.textContent = 'Hide List';
listDiv.style.display = 'block';
} else {
toggleList.textContent = 'Show List';
listDiv.style.display = 'none';
}
});
instead
toggleList.addEventListener('click', () => {
if(listDiv.style.display == 'none') {
toggleList.textContent = 'Hide List';
listDiv.style.display = 'block';
} else {
toggleList.textContent = 'Show List';
listDiv.style.display = 'none';
}
});
why this is happening and what is the relation between them ?
2 Answers

Mathieu St-Vincent
9,244 Pointsvariable.addEventListener(...)
The variable must me a valid html element.
An element can be set with .getElementById or its derivatives.
So for an example:
List.addEventListener('click', () => {})
List is not a variable targeting an element in the DOM.

Mathieu St-Vincent
9,244 PointsThis works as expected, given your example.
addItemButton.addEventListener('click' , () => {...});
It might not work if you add another event listener over it with an error.
Working example: https://jsfiddle.net/5w1yh2yu/
Emre Karakuz
9,162 PointsEmre Karakuz
9,162 PointsYou're right. The variable must me a valid html element. But this is not the answer I'm looking for. Although this block of code is not working because of the incorrect variable :
List.addEventListener('click', () => {...});
This block of code doesn't work too ! (Although everything is written perfectly)
addItemButton.addEventListener('click' , () => {...});