Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial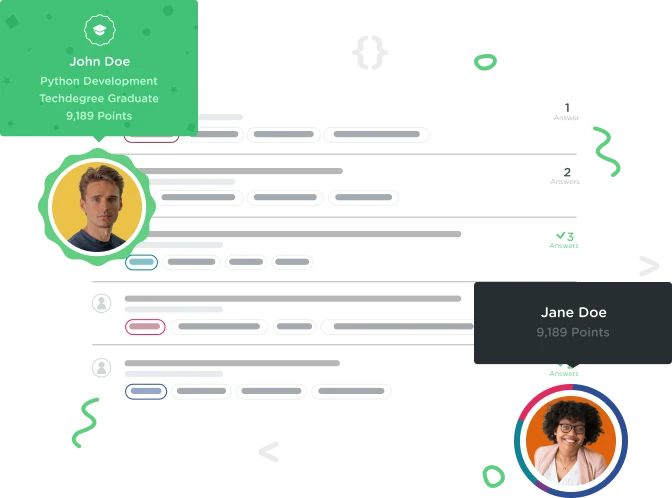
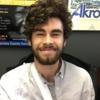
Soren Zeliger
1,282 PointsAppending values from a dictionary to a list
Hi all! As the title suggests, im struggling to add a value from a dictionary to a list. I get an error that seems to say that the value from my dictionary is an optional string while those in the list are strings. Im trying to solve a problem at a point in the collection and controls course where casting optional strings as regular strings (I don't even know if that's a thing) hasnt been discussed, so i dont think im supposed to take that approach, but i cant figure out how else to go about this problem. What I have now is code that declares a list and a simple dictionary, followed by my attempt to append values from the dictionary to the list:
var list: [String] = [] var numDictionary = ["1" : "one", "2" : "two", "3" : "three"] list.append(numDictionary["1"])
and the error that comes up on the append line is "value of optional type 'String?' not unwrapped; did you mean to use '!' or '?'"
Any help would be much appreciated!
1 Answer
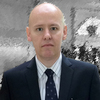
Nathan Tallack
22,159 PointsWhen reading a value from a dictionary using the key in subscript as you are doing the return value will always be an optional. This is so that if the key did not exist the method would return a nil value.
When working with optionals, it is never a good idea to force unwrap them with the !, because your code may not be expecting a nil response and that could cause a crash.
So what we use is an optional binding (if let) to safely unwrap the return of the dictionary value. I have changed your code below to do that.
var list: [String] = []
var numDictionary = [
"1" : "one",
"2" : "two",
"3" : "three"]
if let value = numDictionary["1"] {
list.append(value)
}
So if the key did not exist, or the value of that key was nil, then the if let would skip the code block that does the append and you will not get a crash from the unexpected nil.
If the key did exist and the value was not nil then the value would be assigned to the "value" constant for that code block that follows and we would append that to your list. :)
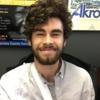
Soren Zeliger
1,282 PointsHey Nathan, thanks a lot for your reply!
This worked, but I don't totally understand why... if let techniques havent been covered yet in the treehouse videos im watching, and the problem where I have to do this (adding a value from a dictionary to a list) is indeed a problem from said track.. so i guess my follow up question is - is there another way to accomplish this?
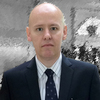
Nathan Tallack
22,159 PointsYeah. I am not sure why with the swift 2 content they are not covering optionals earlier. They covered them pretty early in swift 1.
One thing to remember is when you are told that an optional is not unwrapped you need to unwrap it safely so that you are not surprised with a nil value.
Anything that "could" return a nil value probably does. So think of the dictionary. The key you are looking up might not be there. So you are likely to get a nil back.
When in doubt, you can check the reference guide. If you are in xcode you can option click on your numDictionary variable name, which you are declaring as a dictionary. This will pop up the dictionary reference which lets you read how you work with that type. And of course there is always the swift language guide.
Honestly though, stick at treehouse! Everything I know I learnt from here! So if you keep up the great work you'll get there in the end. :)
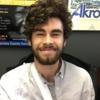
Soren Zeliger
1,282 PointsThank you again so much for your helpful and encouraging reply =) !! I really appreciate it. I will definitely keep in mind your advice. Cheers and see you around!
Ashleigh Nombre
2,308 PointsAshleigh Nombre
2,308 PointsWhen you append you don't need "1" you need [1]