Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial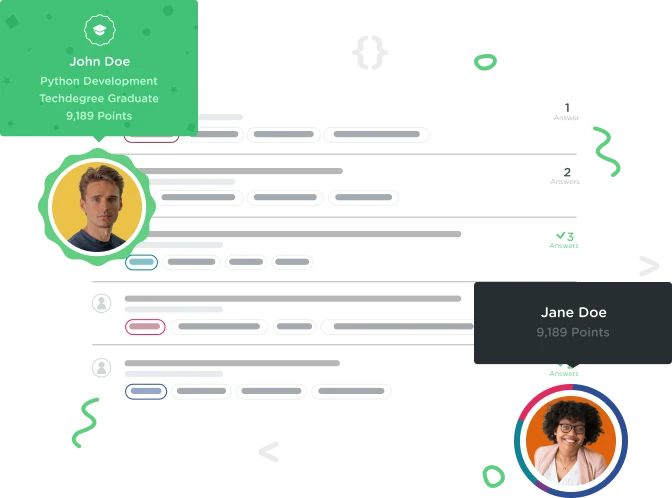

Samuel Trejo
4,563 PointsAppending VS innerHTML
In the video he is using create element to first create the li item, then adding to the ul list with append child, which seems like more work than if you just use innerHTML. Are there any benefits that I am not seeing for using this method?
Seems like my code below is shorter and less work, then having to create new elements each time you want to append a new type of element.
Thanks for the input!
const list = document.querySelector("ul");
const input = document.querySelector("input");
const button = document.querySelector("button");
button.addEventListener("click", () => {
list.innerHTML += `<li>${input.value}</li>`
input.value = ""
})
1 Answer
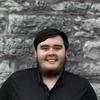
Michael Hulet
47,912 PointsUltimately, the code you posted above is basically equivalent to doing this:
const list = document.querySelector("ul");
const input = document.querySelector("input");
const button = document.querySelector("button");
button.addEventListener("click", () => {
const item = document.createElement("li");
item.innerText = input.value;
list.appendChild(item);
input.value = "";
});
However, doing it the like how my code above does is considered better for a couple reasons. First of all, you don't have to worry about your HTML syntax, as JavaScript will take care of that for you. This makes it impossible for you to accidentally make invalid HTML
Second, in the code in my example, you're allowing JavaScript to communicate with the DOM directly in its own internal representation and better coordinate with the browser, instead of forcing JavaScript to parse your string to figure out what you mean. This leads to very significant performance improvements. According to this jsPerf I made to test, my example runs approximately 590 times faster in Chrome on my machine than using the innerHTML
of the list
. In Safari (which I use most often), it's 1,107 times faster