Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial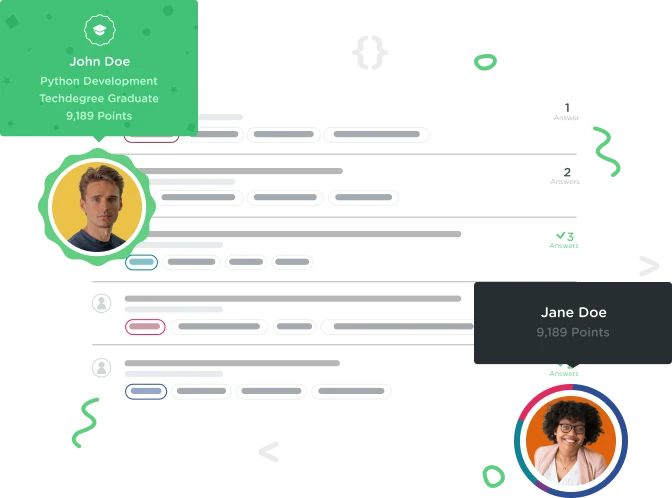

Anthony c
20,907 PointsApplying the Hair Dryer Analogy
How does the hair dryer analogy fit with this diagram?
Which piece of this model (usually) delivers the information about what type of method to use (i.e. what type of outlet prongs are needed). I'm a little foggy on all of this.
Is the adapter letting you group together similar but distinct methods? Then depending on the client's request, it delivers the correct variation of the method to be called?
Or is the adapter modifying things so that the same exact method can be called no matter the input?
I'm also confused as to which of these blocks is happening on the server vs the client
2 Answers

Craig Dennis
Treehouse TeacherHi Anthony c !
The adapter pattern is usually used to wrap an existing class so that it adheres to an interface that another class uses. This is done either at the client or the server, it's a way to make to classes work together.
Here I'll try and explain one in JavaScript. Let's assume we have a method that takes an object, and since it's JavaScript, we're not sure what type is being passed in, all we know by looking at the code is that it requires a render method that doesn't define any parameters.
function displayWebPage(webPage) {
webPage.render();
}
And then let's assume that we have this other Page object that we'd like to display, but it doesn't have a render method.
var Page = function(html) {
this.html = html;
};
Page.prototype.prettyPrint = function() {
document.write(this.html);
};
Let's assume that Page is used in other applications and we don't really want to just add a new method named render
just so it works with the displayWebPage
function. What we can do is wrap it in an adapter like so:
var PageAdapter = function(page) {
this.page = page;
};
PageAdapter.prototype.render = function() {
// The render method simply just calls the underlying method
this.page.prettyPrint();
};
That make sense? It wraps the original method and adapts it to work with the method. Just like the power adapter it takes one input and adapts it to the expected input for the caller.
Hope that helps!

Craig Dennis
Treehouse TeacherHi Anthony c !
The adapter pattern is usually used to wrap an existing class so that it adheres to an interface that another class uses. This is done either at the client or the server, it's a way to make to classes work together.
Here I'll try and explain one in JavaScript. Let's assume we have a method that takes an object, and since it's JavaScript, we're not sure what type is being passed in, all we know by looking at the code is that it requires a render method that doesn't define any parameters.
function displayWebPage(webPage) {
webPage.render();
}
And then let's assume that we have this other Page object that we'd like to display, but it doesn't have a render method.
var Page = function(html) {
this.html = html;
};
Page.prototype.prettyPrint = function() {
document.write(this.html);
};
Let's assume that Page is used in other applications and we don't really want to just add a new method named render
just so it works with the displayWebPage
function. What we can do is wrap it in an adapter like so:
var PageAdapter = function(page) {
this.page = page;
};
PageAdapter.prototype.render = function() {
// The render method simply just calls the underlying method
this.page.prettyPrint();
};
That make sense? It wraps the original method and adapts it to work with the method. Just like the power adapter it takes one input and adapts it to the expected input for the caller.
Hope that helps!
tomasliashuk
8,014 Pointstomasliashuk
8,014 PointsIt might be a bit off topic question, but would the use of a mixin here break our intention not to add new methods?