Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial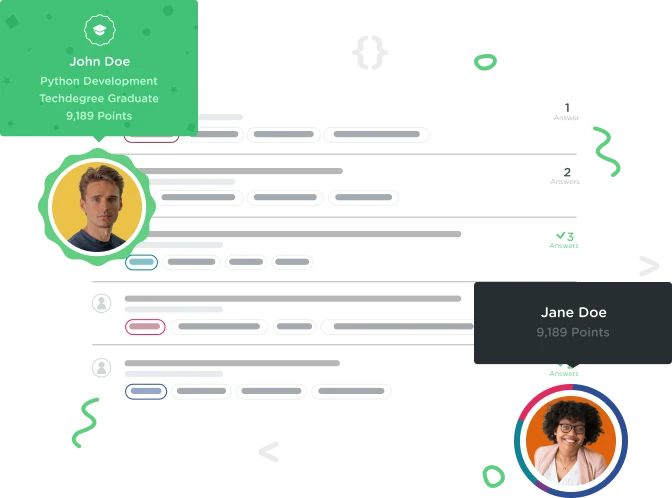
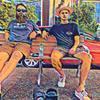
Frederick Bogdanoff
15,338 PointsAppreciate some feed back on what i could've done better.
Hey guys.. This one took me a long time to understand and absorb what I was doing wrong.. However I'm still a bit unhappy: One thing I cant seem to get right is that the incorrect list returns an answer and not a question. Can someone please explain what I'm doing here?
var questions = [
["Who is the creator of Tesla?", "elon musk"],
["Whats the color of the sky?", "blue"],
["I love", "javascript"]
];
var correctAnswers = [];
var wrongAnswers = [];
var correctNums = 0;
var incorrectNums = 0;
function print (message) {
document.write(message);
}
function printCorrect() {
var cList = '<ol>';
print("You answered " + correctNums + " questions correctly");
cList += '<li>' + correctAnswers.join(' <li>') + '</li>' + '</ol>';
document.write(cList);
}
function printWrong() {
var wList = '<ol>';
print("You answered " + incorrectNums + " questions incorrectly");
wList += '<li>' + wrongAnswers.join(' <li>') + '</li>' + '</ol>';
document.write(wList);
}
for (var i = 0; i < questions.length; i += 1) {
var answer = prompt( questions[i][0] );
answer = answer.toLowerCase();
var correct = questions[i][1];
if (correct === answer) {
correctNums += 1;
correctAnswers.push( questions[i][0] );
} else {
incorrectNums += 1;
wrongAnswers.push( questions[i][0] );
}
}
printCorrect();
printWrong();
1 Answer
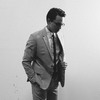
Matt Brock
28,330 PointsHey Frederick, it's working for me. Both the correct list and incorrect list display the question, not the answer. Is it not working for you?
Frederick Bogdanoff
15,338 PointsFrederick Bogdanoff
15,338 PointsWoah... I mustβve been really tired yesterday.. my bad. Its working fine! Is there any other ways I couldβve gone about making the lists without functions?
Matt Brock
28,330 PointsMatt Brock
28,330 PointsI think the way you wrote it works very well. Since both
printCorrect()
andprintWrong()
are quite similar I would suggest abstracting them to one single function, such asprintList()
and accept some arguments. A couple other little things I'd suggest:print()
function and just use the nativedocument.write()
instead.question
to simplify yourfor
loop and make it a little easier to read!variable++;
, which is the exact same thing asvariable += 1;
.Here's what I mean:
Frederick Bogdanoff
15,338 PointsFrederick Bogdanoff
15,338 PointsYou helped me a ton! Thanks!
Matt Brock
28,330 PointsMatt Brock
28,330 PointsHappy to help :)