Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial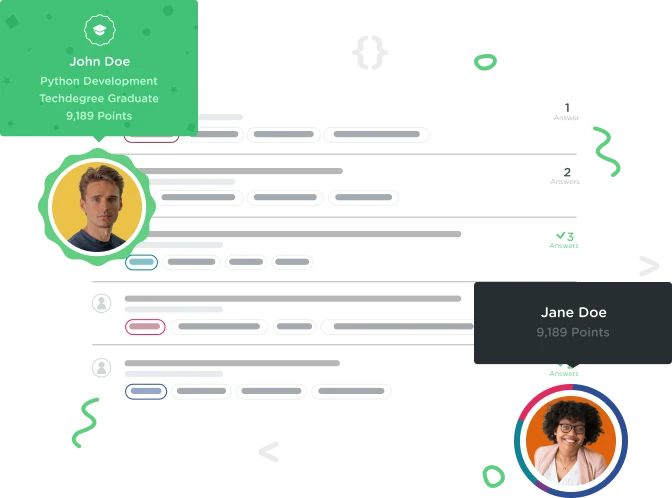
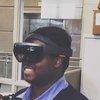
Guled Ahmed
12,806 PointsAre libraries, ones that are imported into our projects, objects that utilize class-level methods?
I've noticed that whenever we import a library in Python, we use the name of the class and then simply use the methods within that class using the dot notation. I recently consolidated my research on classes and discovered, while observing a swift project made by a fellow programmer, that they made a class in which they never instantiated using a variable. Instead what was done was that they typed out the name of the class and used the dot notation immediately to utilize a method like so:
NotificationManager.update();
*NotificationManager was a class in the programmers project.
So I was curious about class-level methods in which you do not have to make a copy of an object and place it in a variable. I'm not sure if I completely understand classes that do not have to be instantiated into a variable ( or is it cloning and object? ). Can anyone explain this and answer my python question. If I am not too clear, please do tell me.
3 Answers

Dan Johnson
40,533 PointsTypically, static methods are methods that don't have access to any instance of a class. Since they don't get passed self
, they can be called directly from the class.
If you want to deal with static or class methods in Python, there are decorators for it:
class MyClass(object):
@staticmethod
def my_func_static():
pass
@classmethod
def my_func_class(cls):
# cls is the type of class that called.
pass
Now for things like importing:
import math
math.isnan(0)
Here you are importing the math module, not a math class. (Though technically speaking a module is a type of class). So when you call isnan, you're not creating a new math class instance, you're just saying, "From the math module that was imported, use isnan".
Say we had a class named MyClass
in a file my_module.py:
class MyClass(object):
def my_method(self):
print("Hello World!")
And then we imported my_module in a separate file and attempted to call my_method like this:
import my_module
my_module.MyClass.my_method()
You will get an error similar to the following:
TypeError: my_method() missing 1 required positional argument: 'self'
Here there was no implicit creation of an instance, and since you weren't dealing with a static or class method, it didn't work. Calling the constructor (__init__
in Python) will fix this:
my_module.MyClass().my_method()
Will print:
Hello World!
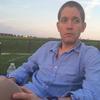
Drew Butcher
33,160 PointsI have notice there are two ways to import files (everything in Python is a class so you could replace the word files with class).
Say I have a file called students.py and it contains a class called Learner. Then I could import by saying:
import student
and then I would have access to the class learner by saying:
learner_one = student.Learner()
Alternatively, I could import the class by saying:
from student import Learner
now I have access to learner without the dot notation
learner_one = Learner()
I hope that helps.
Best, Drew
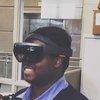
Guled Ahmed
12,806 PointsThis was very helpful. I still wonder about class-level methods. The fact that we don't have to do , as an example: student = student(), but rather we can use "student" (student.Learner()) right out of the box is still something I need to understand. I believe the fact that we can just use a module right out of the box is my question because I'm so use to instantiating things that I haven't seen this kind of usage other than when I started Python. It sort of like the "Math" object in Javascript. Whenever we want to use the Math object, we don't import it in Javascript, we just use Math and then the dot notation (yes we can store it in a variable, but that's more work). I think my actual question is, how is it possible to use these classes in the format -> [Class Name Goes here].[Method goes here] rather than the conventional -> variable = new ( I know python doesn't use 'new') [Class Name Goes Here] Parenthesis Go Here, if I put real parenthesis then this comment might turn into a link :/.
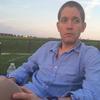
Drew Butcher
33,160 PointsIn python you don't have to declare the variable it get's declared when you define it. You have to be a little careful. For example say you have a list "my_list = ['a','b','c']" then you can can say "my_list[1] = 'e'" and your list would now be: "['a','e','c']"; however, you can NOT say "my_list[3] = 'd'" because my_list[3] doesn't exist.... you would have to use the append method of the list class.... so you would write
my_list.append('d')
Python has a similar math class and if you type
import math
math.pi
then you will get a decimal approximation for pi (3.1415.....); however, you could just type
from math import pi
pi
in this case you now don't have to type the dot notation pi is now an object of your present file or terminal shell.
I'm just a beginner but this is the best of my understanding. :)
Guled Ahmed
12,806 PointsGuled Ahmed
12,806 PointsThis is exactly what I was looking for! Thank you so much.
Drew Butcher
33,160 PointsDrew Butcher
33,160 Pointsthanks
Guled Ahmed
12,806 PointsGuled Ahmed
12,806 PointsAlso, I now know that they are called "static methods" not "class-level methods". Very intriguing.