Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial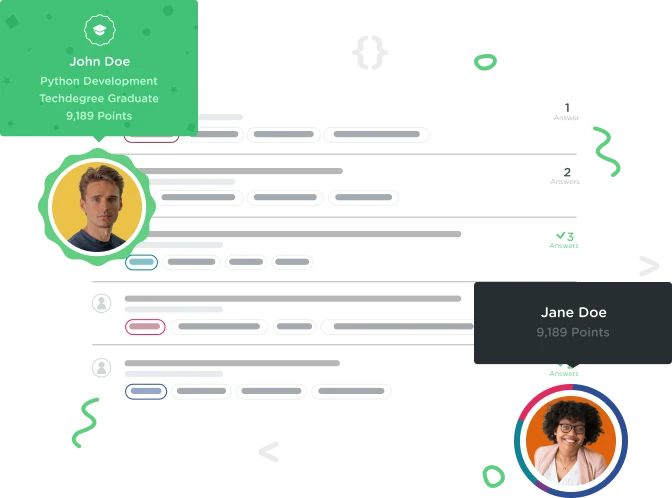

Mathew Gries
7,071 PointsAre the loop counter conditions backwards?
In the video example, you are iterating over the column count for the outer loop, and the row count for the inner loop. Should we not be iterating over the row count for the outer loop, since this loop should be representing each row on the board, and then iterating over the column count in the inner loop, since this should be representing each column in the row?
Maybe this was corrected in a future video, so we will see.
Your code
createSpaces(){
const spaces = [];
for(let x = 0; x < this.columns; x++){
const columns = [];
for(let y = 0; y < this.rows; y++){
const space = new Space(x,y);
columns.push(space);
}
spaces.push(columns);
}
return spaces;
}
What I believe this should be
createSpaces(){
const spaces = [];
for(let x = 0; x < this.rows; x++){
const row = [];
for(let y = 0; y < this.columns; y++){
const space = new Space(x,y);
row.push(space);
}
spaces.push(row);
}
return spaces;
}
1 Answer
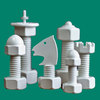
Steven Parker
229,783 PointsI don't think it matters if you loop row-first or column-first; but my personal preference is also rows on the outer loop. However, it does matter that you keep "x" and "y" correctly associated with columns and rows respectively:
for (let y = 0; y < this.rows; y++) { // y for rows
const row = [];
for (let x = 0; x < this.columns; x++) { // x for columns
const space = new Space(x, y); // note: columns come first!
// ...
Mathew Gries
7,071 PointsMathew Gries
7,071 Pointsnot sure that part matters as much. It matters more where they get placed in the Space() constructor. I also think of 2D arrays as rows as the first array index, and columns as the indexes in the rows. This just seemed backwards to me. Maybe it'll play out in the end and make sense why it was it was done this way
Steven Parker
229,783 PointsSteven Parker
229,783 PointsThe order in creating the "space" was my point. In your 2nd example, it should be "
new Space(y, x)
".