Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial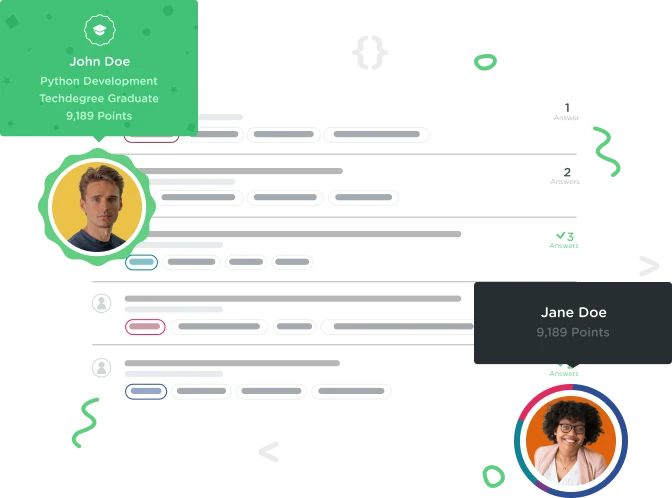

pauladams2
8,399 PointsAre the random numbers stored into an array before being printed to the screen
I am curious, when the code for the while loop is run. The numbers do not print to the screen one by one it waits until the loop has finished and then writes the numbers to the document / browser screen.
So, when you use the while loop to create 10,000 random numbers, are these stored into an array before being written to the browser?
1 Answer

rakan omar
15,066 Pointsyou can do it either way, the following is the code for printing each number seperately:
function getRandom(amount, max) {
for (i=0; i<amount; i++){
console.log(Math.floor(Math.random() * max) + 1);
}
}
and the following is the code for print them in an array once the process is complete:
function getRandom(amount, max) {
var array = [];
for (i=0; i<amount; i++){
array.push(Math.floor(Math.random() * max) + 1);
}
console.log(array);
}
in both function the argument "amount" refers to the amount of random numbers you want and "max" for the maximum random number you want.
for example, getRandom(10, 20); will get you 10 random number ranging from 1 to 20. the first one will print each number seperately as soon as it is generated, the second one will print all numbers in an array once the loop is done to see them in action just paste one in the js console and call it, then do the same for the other