Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial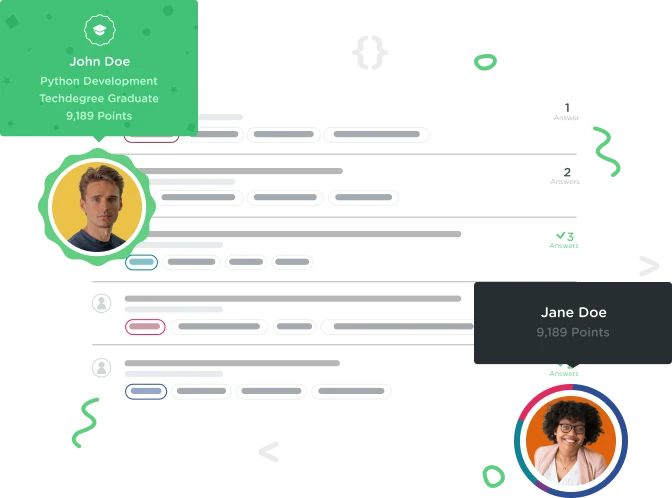
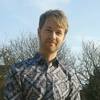
Aaron Watson
2,551 PointsAre variables that are defined inside a function only valid within that function?
I was playing with the code in this video and I was getting confused because I thought we defined message earlier within sayHello() function but if I try and get the content of var message later I get the error "not defined".
Then I was confused by putting message as the argument for debug() can anyone enlighten me? Thanks
var sayHello = function () {
var message = "Hello"
message += " World!"
console.log(message);
}
console.log(message);
sayHello();
var debug = function (message) {
console.log("Debug", message)
};
debug("xyz");
console.log(message);
2 Answers
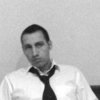
Alex Heil
53,547 Pointshey Aaron Watson , scope in javascript is a pretty interesting topic. by saying this I can highly recommend the new Javascript Basics Course that explains this in more details.
for your particular case, think about it like this: in your function sayHello you created a new variable message - this variable now only lives inside that function.
you could even create another variable with the same name message outside of the function and this would not change the sayHello function in any way. so they're completely seperate and can live side by side without problems.
hope that helps and have a nice day ;)
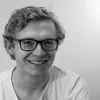
Luke Glazebrook
13,564 PointsHi Aaron!
You are right, variables that are defined inside functions are only used within that function and then they are no longer accessible. If you would like to use a variable that has been declared in a function from outside the function you will have to 'return' the value.
Here is an example of a function returning a value:
var name = "Luke";
function valueReturn(name) {
var greetingMessage = "Hello " + name;
return greetingMessage;
}
You can then access that value easily by setting it equal to a variable. The example below shows this:
var greeting = valueReturn(name)
I hope this helped you out! Please feel free to ask any more questions if you have them!
-Luke
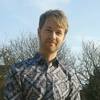
Aaron Watson
2,551 PointsThanks Luke I think I understand it now :-)
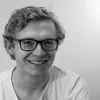
Luke Glazebrook
13,564 PointsAwesome Aaron! Good luck with the rest of your programming!
Aaron Watson
2,551 PointsAaron Watson
2,551 PointsInteresting. So best practice might be to not use it inside and outside even if they can coexist?
Another reason to make tidy code and good names :-)