Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial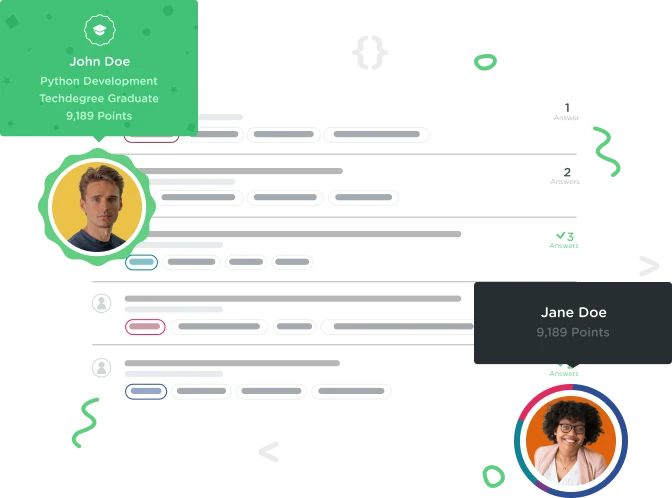

sean tatenda jack
6,127 PointsAren't rules awesome?! I've done all the importing for you here of the rule, but I'd like you to use it in the construc
Aren't rules awesome?! I've done all the importing for you here of the rule, but I'd like you to use it in the constructingLargerThanAlphabetNotAllowed test. It currently throws an exception. Can you use thrown rule I created for you to make sure we are getting the expected message from the assertion?
i think the method is checking whether the exception is throws correctly.so i have changed @Test public void constructingLargerThanAlphabetNotAllowed() throws Exception { new AlphaNumericChooser(27, 10);
to @Test public void constructingLargerThanAlphabetNotAllowed() Exception thrown { new AlphaNumericChooser(27, 10);
but i am still getting errors.
package com.teamtreehouse.vending;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ExpectedException;
import static org.junit.Assert.*;
public class AlphaNumericChooserTest {
private AlphaNumericChooser chooser;
// I added this rule setup code for you, thank me later. ;)
@Rule
public ExpectedException thrown = ExpectedException.none();
@Before
public void setUp() throws Exception {
chooser = new AlphaNumericChooser(26, 10);
}
@Test
public void validInputReturnsProperLocation() throws Exception {
AlphaNumericChooser.Location loc = chooser.locationFromInput("B4");
assertEquals("proper row", 1, loc.getRow());
assertEquals("proper column", 3, loc.getColumn());
}
@Test(expected = InvalidLocationException.class)
public void choosingWrongInputIsNotAllowed() throws Exception {
chooser.locationFromInput("WRONG");
}
@Test(expected = InvalidLocationException.class)
public void choosingLargerThanMaxIsNotAllowed() throws Exception {
chooser.locationFromInput("B52");
}
@Test
public void constructingLargerThanAlphabetNotAllowed()exception thrown {
new AlphaNumericChooser(27, 10);
}
}
package com.teamtreehouse.vending;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class AlphaNumericChooser extends AbstractChooser {
private int ALPHA_FIRST = (int) 'A';
private int ALPHA_LAST = (int) 'Z';
public AlphaNumericChooser(int maxRows, int maxColumns) {
super(maxRows, maxColumns);
int maxAllowedAlpha = (ALPHA_LAST - ALPHA_FIRST) + 1;
if (maxRows > maxAllowedAlpha) {
throw new IllegalArgumentException("Maximum rows supported is " + maxAllowedAlpha);
}
}
@Override
public Location locationFromInput(String input) throws InvalidLocationException {
Pattern pattern = Pattern.compile("^(?<row>[a-zA-Z]{1})(?<column>[0-9]+)$");
Matcher matcher = pattern.matcher(input);
if (!matcher.matches()) {
throw new InvalidLocationException("Invalid buttons");
}
int row = inputAsRow(matcher.group("row"));
int column = inputAsColumn(matcher.group("column"));
return new Location(row, column);
}
private int inputAsRow(String rowInput) {
rowInput = rowInput.toUpperCase();
return (int) rowInput.charAt(0) - ALPHA_FIRST;
}
private int inputAsColumn(String columnInput) {
int columnAsInt = Integer.parseInt(columnInput);
return columnAsInt - 1;
}
}
package com.teamtreehouse.vending;
public abstract class AbstractChooser {
private final int maxRows;
private final int maxColumns;
public class Location {
private final int row;
private final int column;
protected Location(int row, int column) throws InvalidLocationException {
if (row > maxRows || column > maxColumns) {
throw new InvalidLocationException("Invalid Location");
}
this.row = row;
this.column = column;
}
public int getRow() {
return row;
}
public int getColumn() {
return column;
}
}
public AbstractChooser(int maxRows, int maxColumns) {
this.maxRows = maxRows;
this.maxColumns = maxColumns;
}
public abstract Location locationFromInput(String input) throws InvalidLocationException;
}
package com.teamtreehouse.vending;
public class InvalidLocationException extends Exception {
public InvalidLocationException(String s) {
super(s);
}
}
2 Answers
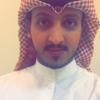
Fahad Mutair
10,359 Points@Test
public void constructingLargerThanAlphabetNotAllowed() throws Exception {
thrown.expect(IllegalArgumentException.class);
thrown.expectMessage("Maximum rows supported is 26");
new AlphaNumericChooser(27, 10);
}
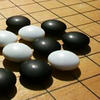
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsNo, you don't change it to "exception thrown", it's not even valid java syntax. You need to use an annotation similar to the two previous tests, like "@Test(expected = InvalidLocationException.class)" (but with the correct exception class instead). You should probably watch the video again. Programming is unforgiving with inexact syntax. It's important to pay attention to the details.
sean tatenda jack
6,127 Pointssean tatenda jack
6,127 Pointsbest answer
Oshedhe Munasinghe
8,108 PointsOshedhe Munasinghe
8,108 PointsAhha thank you!! I accidentally called InvalidLocationException which is wrong!! Good job! :D