Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial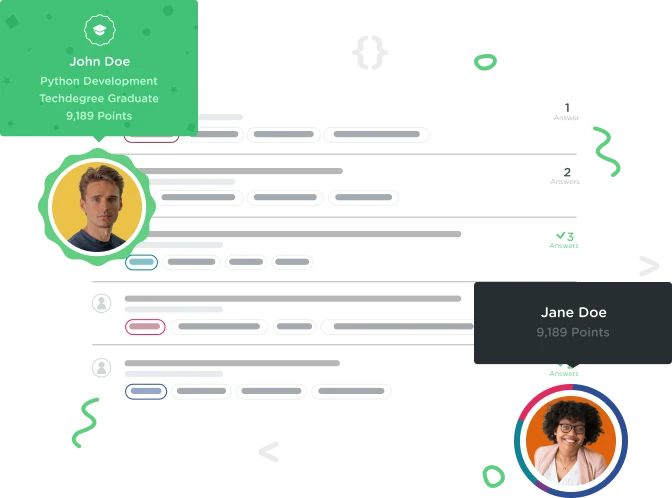

praveen gudimetla
464 PointsAren't rules awesome?! I've done all the importing for you here of the rule, but I'd like you to use it in the construct
Hi,
I was not sur how to use Thrown
package com.teamtreehouse.vending;
import org.junit.Before; import org.junit.Rule; import org.junit.Test; import org.junit.rules.ExpectedException;
import static org.junit.Assert.*;
public class AlphaNumericChooserTest {
private AlphaNumericChooser chooser;
// I added this rule setup code for you, thank me later. ;)
@Rule
public ExpectedException thrown = ExpectedException.none();
@Before
public void setUp() throws Exception {
chooser = new AlphaNumericChooser(26, 10);
}
@Test
public void validInputReturnsProperLocation() throws Exception {
AlphaNumericChooser.Location loc = chooser.locationFromInput("B4");
assertEquals("proper row", 1, loc.getRow());
assertEquals("proper column", 3, loc.getColumn());
}
@Test(expected = InvalidLocationException.class)
public void choosingWrongInputIsNotAllowed() throws Exception {
chooser.locationFromInput("WRONG");
}
@Test(expected = InvalidLocationException.class)
public void choosingLargerThanMaxIsNotAllowed() throws Exception {
chooser.locationFromInput("B52");
}
@Test
public void constructingLargerThanAlphabetNotAllowed() throws Exception {
new AlphaNumericChooser(27, 10);
}
}
we already have the required methodd here right as below? @Test public void constructingLargerThanAlphabetNotAllowed() throws Exception { new AlphaNumericChooser(27, 10); }
package com.teamtreehouse.vending;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ExpectedException;
import static org.junit.Assert.*;
public class AlphaNumericChooserTest {
private AlphaNumericChooser chooser;
// I added this rule setup code for you, thank me later. ;)
@Rule
public ExpectedException thrown = ExpectedException.none();
@Before
public void setUp() throws Exception {
chooser = new AlphaNumericChooser(26, 10);
}
@Test
public void validInputReturnsProperLocation() throws Exception {
AlphaNumericChooser.Location loc = chooser.locationFromInput("B4");
assertEquals("proper row", 1, loc.getRow());
assertEquals("proper column", 3, loc.getColumn());
}
@Test(expected = InvalidLocationException.class)
public void choosingWrongInputIsNotAllowed() throws Exception {
chooser.locationFromInput("WRONG");
}
@Test(expected = InvalidLocationException.class)
public void choosingLargerThanMaxIsNotAllowed() throws Exception {
chooser.locationFromInput("B52");
}
@Test
public void constructingLargerThanAlphabetNotAllowed() throws Exception {
new AlphaNumericChooser(27, 10);
}
}
package com.teamtreehouse.vending;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class AlphaNumericChooser extends AbstractChooser {
private int ALPHA_FIRST = (int) 'A';
private int ALPHA_LAST = (int) 'Z';
public AlphaNumericChooser(int maxRows, int maxColumns) {
super(maxRows, maxColumns);
int maxAllowedAlpha = (ALPHA_LAST - ALPHA_FIRST) + 1;
if (maxRows > maxAllowedAlpha) {
throw new IllegalArgumentException("Maximum rows supported is " + maxAllowedAlpha);
}
}
@Override
public Location locationFromInput(String input) throws InvalidLocationException {
Pattern pattern = Pattern.compile("^(?<row>[a-zA-Z]{1})(?<column>[0-9]+)$");
Matcher matcher = pattern.matcher(input);
if (!matcher.matches()) {
throw new InvalidLocationException("Invalid buttons");
}
int row = inputAsRow(matcher.group("row"));
int column = inputAsColumn(matcher.group("column"));
return new Location(row, column);
}
private int inputAsRow(String rowInput) {
rowInput = rowInput.toUpperCase();
return (int) rowInput.charAt(0) - ALPHA_FIRST;
}
private int inputAsColumn(String columnInput) {
int columnAsInt = Integer.parseInt(columnInput);
return columnAsInt - 1;
}
}
package com.teamtreehouse.vending;
public abstract class AbstractChooser {
private final int maxRows;
private final int maxColumns;
public class Location {
private final int row;
private final int column;
protected Location(int row, int column) throws InvalidLocationException {
if (row > maxRows || column > maxColumns) {
throw new InvalidLocationException("Invalid Location");
}
this.row = row;
this.column = column;
}
public int getRow() {
return row;
}
public int getColumn() {
return column;
}
}
public AbstractChooser(int maxRows, int maxColumns) {
this.maxRows = maxRows;
this.maxColumns = maxColumns;
}
public abstract Location locationFromInput(String input) throws InvalidLocationException;
}
package com.teamtreehouse.vending;
public class InvalidLocationException extends Exception {
public InvalidLocationException(String s) {
super(s);
}
}
4 Answers

praveen gudimetla
464 Pointsi was watching video again.
i wonder when addding Doritos and Cheetos at 4:21 min throws exception? i am trying to relate that with my challenge. exception thrown: test passes how if exception thrown test passes?
also how exception not thrown means test does not pass ? please elaborate

praveen gudimetla
464 Pointsplease advise

praveen gudimetla
464 PointsI found one good video on rules https://www.youtube.com/watch?v=utAtA0qqL-I

praveen gudimetla
464 Pointsi see after 10 minutes of 12 minutes total video it was explained similar to video 9 min concept before. i will try to get same message and instead of exception in @Test expected i will write inside method body as speaker did
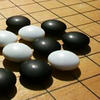
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsYes, the method is already there, but it's not working. Try clicking preview and you'll see the test does not pass. Here the method is checking whether the exception is thrown correctly. So the wanted behavior is:
- exception thrown: test passes
- exception not thrown: test does not pass At the moment the test considers the exception like a sign that something didn't work. You must use the created rule to make sure the reverse happens, and the thrown exception is considered like the normal, expected behavior. Now that you understand what your goal is, have another look at the video, it shows clearly how to do this.