Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial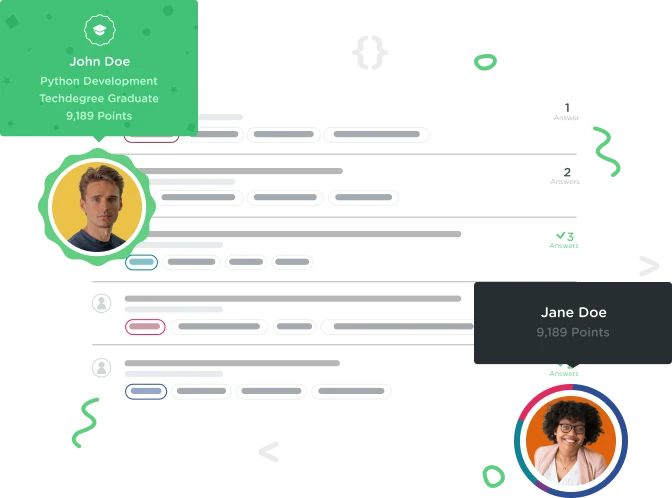
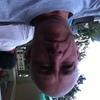
Stuart McIntosh
Python Web Development Techdegree Graduate 22,874 PointsArgon2 error
hi there, i am running through the Flask API course and get the following error when trying to post a new course with authorisation via postman
argon2.exceptions.VerificationError: Decoding failed
1 Answer

Bleza Takouda
5,129 PointsI came across the same error. But my case was a bit different since I did the POST to the API using CLI. Proper encoding option is required when using CLI to interact with the API.
Mark Ramos
19,209 PointsMark Ramos
19,209 PointsJust posting here to say I got the same error.