Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial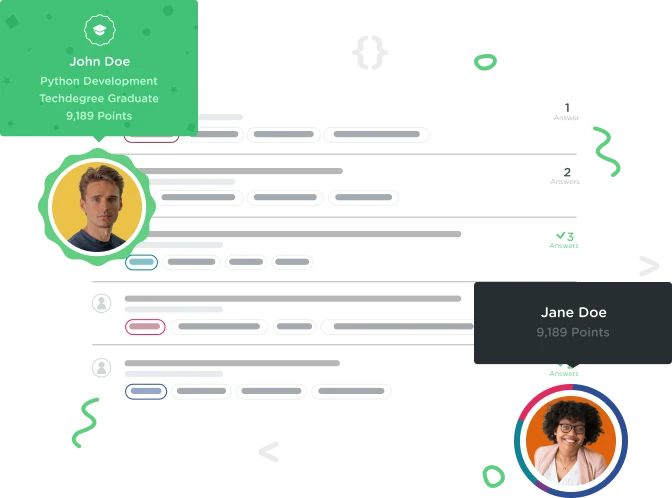

orange sky
Front End Web Development Techdegree Student 4,945 Pointsargs 1 assignment
Hello!
I am able to get somewhat to output the following int the console: "Hello Michael, my name is Andrew and I am in a awesome mood!"
But there is a ':)' in this array and it does not appear in my output: var args1 = ["Michael", "awesome", ":)"];
Can someone please give me a nudge in the right direction. Also, I am not sure if the my code set up is ok.
thanks
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Objects</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Objects: Call and Apply</h2>
<script>
var genericGreet = function(name, mood) {
name = name || "you";
mood = mood || "good";
return "Hello " + name + ", my name is " + this.name +
" and I am in a " + mood + " mood!";
}
var andrew = {
name: "Andrew",
greeting: genericGreet
}
var args1 = ["Michael", "awesome", ":)"];
console.log( andrew.greeting.apply(andrew, args1));
// var greeting = genericGreet;
</script>
</body>
</html>
4 Answers
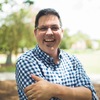
Dave McFarland
Treehouse TeacherYou can access the third item in the args1
array in a couple of different ways. When args1
is applied to the genericGreet method
, the first two array items -- "Michael"
and "awesome"
-- are put into the parameters name
and mood
. You can add a third parameter like this:
var genericGreet = function (name, mood, emoticon) {
}
You could then access that third parameter using the emoticon
variable within the function.
Also, the genericGreet function receives the args1
argument in an arguments
object -- this is a special object in JavaScript functions which contains an array-like object of all the arguments passed to the function. So you could access each item passed to the genericGreet function:
var genericGreet = function ( ) {
console.log(arguments[0]); // "Michael"
console.log(arguments[]); // "awesome"
console.log(arguments[2]); // ":)"
}

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello Dave,
I have added the parameter emoticon into the genericGreeting(), just like you recommended, but I am still getting the same output in the console (only the emoticon does not show) my console shows: "Hello Michael, my name is Andrew and I am in a awesome mood!"
thank you for your help!!!!
Here is the adjusted code:
<!DOCTYPE html> <html lang="en"> <head> <title> JavaScript Foundations: Objects</title> <style> html { background: #FAFAFA; font-family: sans-serif; } </style> </head> <body> <h1>JavaScript Foundations</h1> <h2>Objects: Call and Apply</h2> <script> var genericGreet = function(name, mood, emoticon) { name = name || "you"; mood = mood || "good"; return "Hello " + name + ", my name is " + this.name + " and I am in a " + mood + " mood!"; } var args1 = ["Michael", "awesome", ":)"];
var andrew = {
name: "Andrew",
greet: genericGreet
}
console.log( andrew.greet.apply( andrew, args1));
</script>
</body> </html>

orange sky
Front End Web Development Techdegree Student 4,945 PointsOk, I realized that I had not passed the value of emoticon into my return function. This code below outputs the correct sentence, which is :
"Hello Michael, my name is Andrew and I am in a awesome mood!:)"
but I still did not pass, hmm I am not sure anymore.
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Objects</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Objects: Call and Apply</h2>
<script>
var genericGreet = function(name, mood, emoticon) {
name = name || "you";
mood = mood || "good";
return "Hello " + name + ", my name is " + this.name +
" and I am in a " + mood + " mood!" + emoticon;
}
var args1 = ["Michael", "awesome", ":)"];
var andrew = {
name: "Andrew",
greet: genericGreet
}
console.log( andrew.greet.apply( andrew, args1));
</script>
</body>
</html>

orange sky
Front End Web Development Techdegree Student 4,945 PointsI understand arguments now. Thanks!!!!
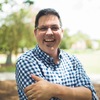
Dave McFarland
Treehouse TeacherGreat! Glad I could help.
orange sky
Front End Web Development Techdegree Student 4,945 Pointsorange sky
Front End Web Development Techdegree Student 4,945 PointsI see. Arguments is an ojects that allows us to view the values stored in an array. OK, may, I please see the entire code with 'arguments' in the console. This is my first time to come across this object. Thanks!!!!!
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse Teacher