Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial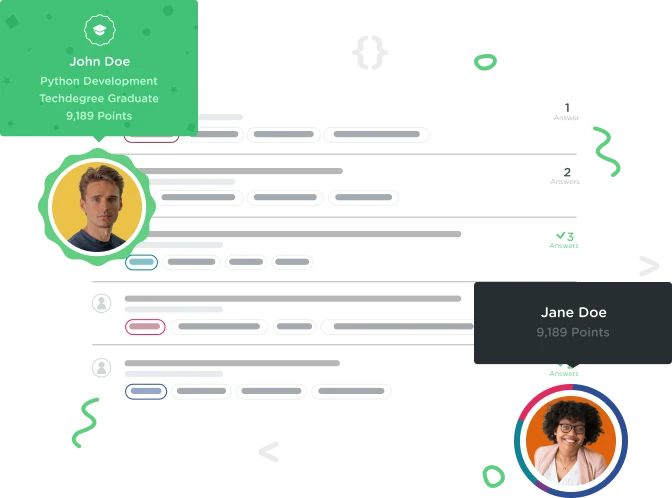

Freddy Venegas
1,226 PointsArgument in def add_to_list(): is it necessary?
When the def add_to_list(new_item):
function was created I noticed that it works with or without the argument 'new_item' why is that? So, if I run my script like this,
def add_to_list(new_item):
# add new items to list
shopping_list.append(new_item)
print("Added {}. List now has {} of items.".format(new_item, len(shopping_list)))
# while loop would be here
...
add_to_list(new_item)
or like this,
def add_to_list():
# add new items to list
shopping_list.append(new_item)
print("Added {}. List now has {} of items.".format(new_item, len(shopping_list)))
# while loop would be here
...
add_to_list()
both work just fine. So is passing the argument really necessary?
5 Answers
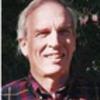
jcorum
71,830 PointsFreddy, when I run your second version I get this:
Traceback (most recent call last):
File "test.py", line 5, in add_to_list
shopping_list.append(new_item)
NameError: name 'new_item' is not defined

Idan Melamed
16,285 PointsIf I recall correctly it's a matter of scope.
You might want to try reading the next link to understand it better: http://eli.thegreenplace.net/2011/05/15/understanding-unboundlocalerror-in-python

Freddy Venegas
1,226 PointsThank you Idan for this link. I shall have a read and familiarize myself as much as I can.
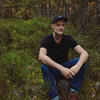
Simon Dein
4,772 PointsI have the same question - and my code runs fine without taking "new_item" as an argument
# making shopping list
shopping_list = []
# help function
def show_help():
print("""
Press 'enter' to add item to shopping list
Enter 'DONE' to stop adding items and show final shopping list
Enter 'HELP' to get up this screen
Enter 'SHOW' to show what is currently on your shopping list
""")
# add items to shopping list
def add_new_item():
shopping_list.append(new_item)
print("{} added. {} items on list.".format(new_item, len(shopping_list)))
# show list function
def show_list():
print("Here's your list:")
for item in shopping_list:
print(item)
show_help()
# ask for new additions to shopping list
while True:
new_item = input("> ")
# if done - loop will break
if new_item == 'DONE':
break
# help for showing instructions+commands
elif new_item == 'HELP':
show_help()
continue
# show what's currently on shopping list
elif new_item == 'SHOW':
print(shopping_list)
continue
add_new_item()
# print out shopping list
show_list()

Freddy Venegas
1,226 PointsI believe in this case it will work without the argument because you are not calling it in def
if you were to pass the argument in the function and then try to call the function without the argument you would get something like this
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: add_new_item() takes exactly 1 argument (0 given)
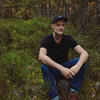
Simon Dein
4,772 PointsAllrighty. I would would really like a basic explaination on why he'd want to put in the argument :)

Freddy Venegas
1,226 PointsI'm not 100% sure myself.

Idan Melamed
16,285 PointsHi Simon,
I think you program works because you created the variable shopping_list outside of a function at the beginning of your code, and that tells Python that it's a global variable.
Usually all your code will be written inside functions, then you will have to pass the variables you want to functions that need them.
Freddy Venegas
1,226 PointsFreddy Venegas
1,226 PointsYou are right, I must have been running it with the argument and didn't realize. My mistake! Thank you for your looking into and response to this non-issue.