Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial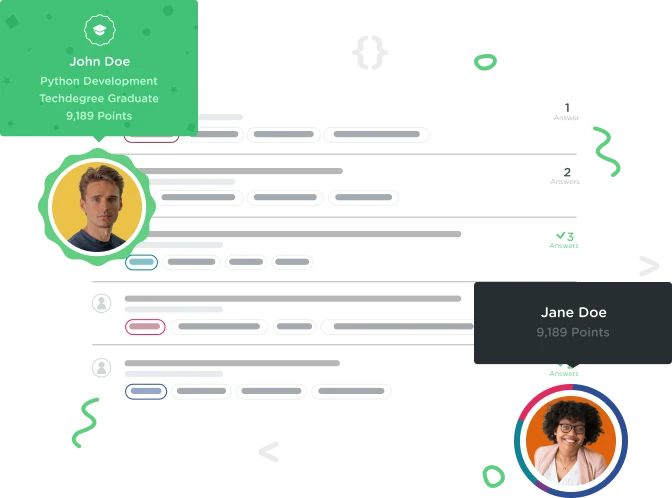

John Perry
Full Stack JavaScript Techdegree Graduate 41,012 PointsArgument type '(HourlyEmployee).Type' does not conform to expected type 'Payable'
Here's my code:
import UIKit
import Foundation
protocol Payable {
func pay() -> (basePay: Double, benefits: Double, deductions: Double, vacation: Int)
}
enum EmployeeType {
case Manager
case NotManager
}
class Employee {
let name: String
let address: String
let startDate: NSDate
let type: EmployeeType
var department: String?
var reportsTo: String?
init(fullName: String, employeeAddress: String, employeeStartDate: NSDate, employeeType: EmployeeType) {
self.name = fullName
self.address = employeeAddress
self.startDate = employeeStartDate
self.type = employeeType
}
}
func payEmployee(employee: Payable) {
let paycheck = employee.pay()
}
class HourlyEmployee: Employee,Payable {
var hourlyWage: Double = 15.00
var hoursWorked: Double = 0
let availableVacation = 0
func pay() -> (basePay: Double, benefits: Double, deductions: Double, vacation: Int) {
return (hourlyWage * hoursWorked, 0, 0, availableVacation)
}
}
let hourlyEmployee = HourlyEmployee(fullName: "John", employeeAddress: "none", employeeStartDate: NSDate(), employeeType: .NotManager)
payEmployee(HourlyEmployee)
1 Answer
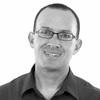
David Papandrew
8,386 PointsHi John,
There is a typo in your last line of code it should read:
payEmployee(hourlyEmployee)
The difference being that in your original code you wrote "HourlyEmployee". Instead, you want to pass the hourlyEmployee constant (with a lowercase h) to the payEmployee func.
Hope that helps.