Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial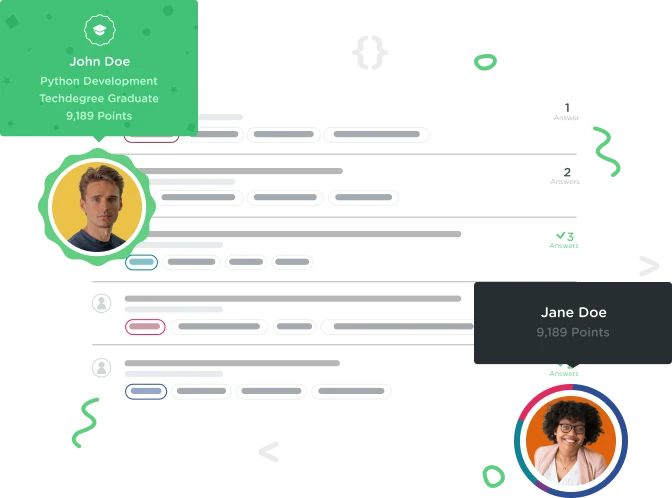
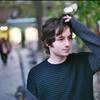
Atıl Kurtulmuş
4,945 PointsArguments and booleans in a function
I have no past coding experience and I started learning with python. Treehouse is a great website and I love it. I believe I'm making some progress but there is one thing, so far, I cannot get a grasp of. Why do some specific arguments and booleans go into a function?In the code below, for example, why do we have to inclue list names as arguments in functions like draw and get_guess and done boolean into play function?
import random
import os
import sys
words = ["elma","armut","karpuz"]
def draw(bad_guess, good_guess, word):
print("Yanlış tahmin sayısı: {}/10".format(len(bad_guess)))
print("")
for letter in bad_guess:
print(letter, end=" ")
print("")
for letter in word:
if letter in good_guess:
print(letter, end=" ")
else:
print("_", end=" ")
print("")
def get_guess(bad_guess, good_guess):
while True:
guess = input("Bir harf tahmin et: ").lower()
if len(guess) != 1:
print("Sadece tek bir harf tahmin edebilirsin")
elif guess in bad_guess or guess in good_guess:
print("Bu harfi daha önce tahmin ettin")
elif not guess.isalpha():
print("Sadece harf tahmin edebilirsin")
else:
return guess
def play(done):
word = random.choice(words)
bad_guess = []
good_guess = []
while True :
draw(bad_guess, good_guess, word)
guess = get_guess(bad_guess, good_guess)
if guess in word:
good_guess.append(guess)
found = True
for letter in word:
if letter not in good_guess:
found = False
if found:
print("Kazandın!")
print("Doğru kelime: {}".format(word))
done = True
else:
bad_guess.append(guess)
if len(bad_guess) == 10:
draw(bad_guess,good_guess,word)
print("Kaybettin.")
print("Doğru kelime: {}".format(word))
done = True
if done:
tekrar = input("Tekrar oyna E/H").lower()
if tekrar != h:
return play(done= False)
else:
sys.exit()
def welcome():
başla = input("Başlamak için Enter'a ya da çıkmak için Q'ya bas").lower()
if başla == "q":
sys.exit
else:
return True
done = False
while True:
welcome()
play(done)
1 Answer
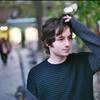
Atıl Kurtulmuş
4,945 PointsMany thanks for your answer! You made it very clear. I think i'm starting to understand how building a function works after a lot of messing around with pycharm. Seeing what the function really does in practice after you write it helps a lot for understanding the basics.
Zach Anderson
3,691 PointsZach Anderson
3,691 PointsIf I understand correctly what you're asking about is the difference between local and global variables and function parameters. I believe this topic gets covered later in the course (although I'm not there yet) but here's a super quick version:
(Real quick, I should let you know that boolean is just a datatype. Variables can be integers, floats, strings, booleans, or a whole bunch of other things but I don't think it exactly ties in to your main question.)
A global variable is any variable defined outside of a function. In your code the variable words is global. That means whenever you use the variable words it will always use that same variable.
A local variable is created temporarily each time the function is run. So when you defined the function draw with three parameters (which is data that you give to draw() to use as local variables) the timeline sorta looks like this:
def function_name(parameter1, parameter2, etc.):
Here's another way of looking at it:
draw('value1', 23, 'yay!')
will run the function code that you defined in draw(). When it runs that code it will pop 'value1' into a local variable called bad_guess and 23 will get put into a local variable called good_guess and 'yay!' will be put into word. Basically doing this:
The beauty of this is it lets you run code where you don't know what bad_guess is, or in this case you're running code where bad_guess will be changing, so you can't just write in bad_guess = 'value1'
*(unless you've got a global variable named bad_guess as well, but I wouldn't recommend doing that!)
...hopefully this explanation helps somewhat... I tried to make it simpler but I may just be more confusing ? Like I said I haven't gotten to global/local variables yet (though apparently I've some passing familiarity) so I'm hoping what I said is accurate.
Cheers, -Zach