Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial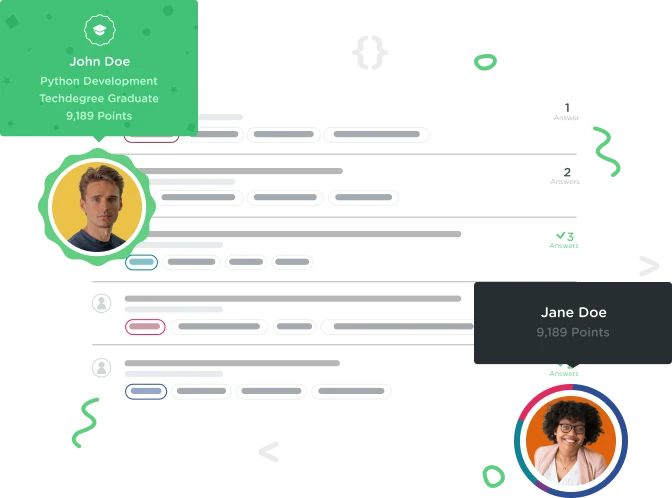

Michael Apps
4,907 PointsArithmetic Calculator
Hi
I am having a go at the Arithmetic Calculator exercise at the end of the C# Basics course. I am really struggling to figure out how it is that you can have the user input the operator and then use it in the calculation. I've google'd to no avail.
Any help would be appreciated. Thank you.
3 Answers
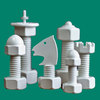
Steven Parker
229,783 PointsYou might not use it directly. But if you were to input an operator symbol as a string, you could then test that string against a set of literal strings that represent operations your program can do, and perform that operation when you have a match.
Does that help?

Michael Apps
4,907 PointsHi, thanks for the reply Steven. Not really, sorry (just started learning a few days ago).
Here is where I am so far. I know things have been repeated where there is probably a much more efficient way of doing it but I'm just trying to use what has been taught in the course so far.
I am stuck figuring out how to make var b the operator and then use it to get runningTotal. Apologies if I am going about it completely wrong .
using System;
namespace MikeCode
{
class Arithmatic
{
static void Main()
{
var runningTotal = 0.0;
while(true)
{
//Prompt the user for a number.
Console.WriteLine("Enter a number");
var a = Console.ReadLine();
if (a.ToLower() == "quit")
{
break;
}
else
{
double.Parse(a);
}
//Prompt the user for an operation (+ - / *).
Console.WriteLine("Enter an operator");
var b = Console.ReadLine();
if (b.ToLower() == "quit")
{
break;
}
//Prompt the user for another number.
Console.WriteLine("Enter another number");
var c = Console.ReadLine();
if (c.ToLower() == "quit")
{
break;
}
else
{
double.Parse(c);
}
//Perform the operation.
runningTotal = a b c;
//Print the result to the screen.
Console.WriteLine(runningTotal);
//Repeat until the user types in βquitβ at any of the prompts.
}
Console.WriteLine("GoodBye!");
}
}
}
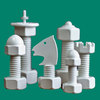
Steven Parker
229,783 PointsYou'll need an assignment to save the results of your numeric conversions so you can use them later:
a = double.Parse(a);
Be sure to something similar with "c". Then applying the hint I gave you before:
//Perform the operation.
if (b == '+') {
runningTotal = a + c;
}
else if (b == '-') {
runningTotal = a - c;
}
// etc...

Michael Apps
4,907 PointsThank you Steven, that's a big help.

Charlie Harcourt
8,046 PointsHi, May I ask why you're doing a double.Parse(a)? I don't quite understand that part.
Thanks.
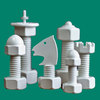
Steven Parker
229,783 PointsThe "double.Parse
" function converts a string made up of digits (and optional decimal point) into a number. This is necessary to be able to perform math on it.