Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial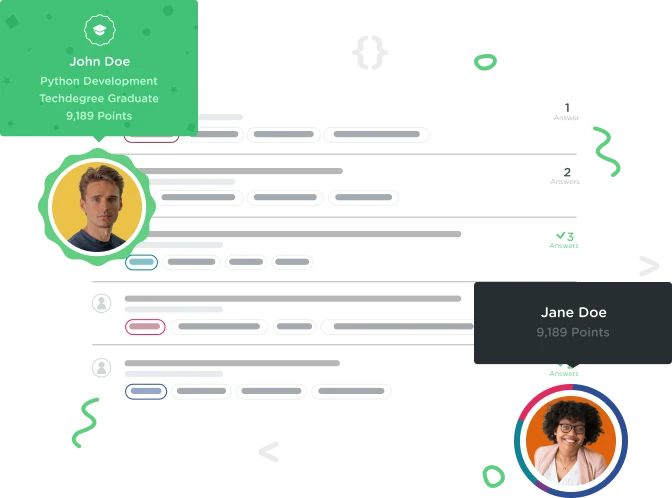
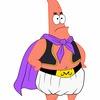
<noob />
17,062 PointsArithmetic Calculator challenge problem, I cant exit the program for some reason.
Hi, This is my code:
using System;
namespace TreeHouse.Calculator
{
class Calculator
{
static void Main()
{
var totalResult = 0.0;
while(true)
{
try
{
Console.WriteLine("Please Enter a number: ");
string entry = Console.ReadLine();
var number = double.Parse(entry);
if(entry.ToLower() == "quit") {
break;
}
Console.WriteLine("Please enter an operation [+ - / * ]: ");
var entry2 = Console.ReadLine();
if(entry2.ToLower() == "quit") {
break;
}
Console.WriteLine("Please enter another number: ");
string entry3 = Console.ReadLine();
var number2 = double.Parse(entry3);
if(entry3.ToLower() == "quit") {
break;
}
if(entry2 == "+")
{
totalResult = number + number2;
}
else if(entry2 == "-")
{
totalResult = number - number2;
}
else if(entry2 == "*")
{
totalResult = number * number2;
}
else if(entry2 == "/")
{
totalResult = number / number2;
}
Console.WriteLine("the result is: {0}", totalResult);
}
catch(FormatException) {
Console.WriteLine("Your input is invalid, Please try again!..");
continue;
}
}
}
}
}
when i try to exit the program by typing 'quit', i get the messege from my exception, I checked alot of times and i can't find the problem why my 'if' statment are not working..
another problem i have is when i type a string or a number in a operator prompt it pass and in result the "final result" become 0.. any ideas how to fix this?
:D?
1 Answer
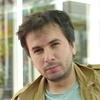
Erik Gabor
6,427 PointsThe reason are the following codes
Console.WriteLine("Please Enter a number: ");
string entry = Console.ReadLine();
var number = double.Parse(entry);
if(entry.ToLower() == "quit") {
break;
}
//....
Console.WriteLine("Please enter another number: ");
string entry3 = Console.ReadLine();
var number2 = double.Parse(entry3);
if(entry3.ToLower() == "quit") {
break;
}
These codes are contained in a try catch block. So when you enter "quit" you already caused a format exception and in the catch block you have continue which sends to the beginning of the while loop. The solution would have been to call the parse after checking "quit". You did this by restructuring the code in the second example.
<noob />
17,062 Points<noob />
17,062 Points****** UPDATE ******
I somehow tried to get all this code outside the 'try' check
It's working now but i dont fully understand why i had to get this code outside of the "TRY" check.
and i still didnt fixed the other problem i mentioned before.