Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial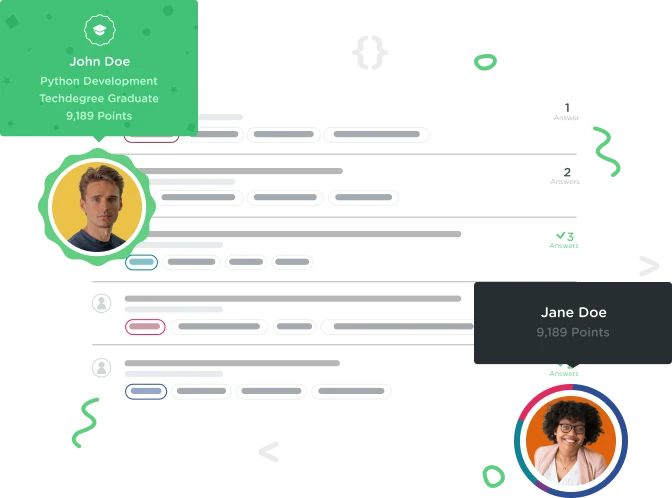

Benjamin Stano
2,742 PointsArray Flattening Methods Challenge Question
I am having trouble figuring out how to flatten the strings using only the reduce method. I tried to emulate the method the teacher in the video used for the similar problem, where he called '.map' twice in once {{e.g. hobbies = customers.map(customers => customer.personal.map(person => person.hobbies))}} and then calling reduce on it. But it doesn't seem to work here. I have tried looking at other resources but I feel very lost right now.
const customers = [
{
name: "Tyrone",
personal: {
age: 33,
hobbies: ["Bicycling", "Camping"]
}
},
{
name: "Elizabeth",
personal: {
age: 25,
hobbies: ["Guitar", "Reading", "Gardening"]
}
},
{
name: "Penny",
personal: {
age: 36,
hobbies: ["Comics", "Chess", "Legos"]
}
}
];
let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
// Write your code below
hobbies = customers.reduce((arr, hobby) => arr.concat(hobby), []);
console.log(hobbies);
1 Answer
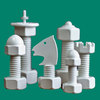
Steven Parker
231,268 PointsYou have the right idea to use the spread operator to combine arrays, but you don't need to use "map" if you reference the member of the object that stores the hobbies directly in the callback function.
You can't call "cust.personal.map" anyway, because "map" is an array method, but "cust.personal" is an object.

Benjamin Stano
2,742 PointsOoh, that makes a lot of sense. But, I'm not sure how I would reference the object member in the callback function. I feel like the video in this course didn't tell me a lot about the spread operator or the reduce function. Something like?:
hobbies = customers.reduce((arr,person) => [...arr,...person.personal.hobbies], []);
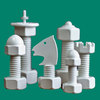
Steven Parker
231,268 PointsExactly like that, good job! Use that code and pass the challenge.
Benjamin Stano
2,742 PointsBenjamin Stano
2,742 PointsCode I tried to emulate: const books = users .map(user => user.favoriteBooks.map(book => book.title)) .reduce((arr, titles) => [...arr, ...titles],[]);
Code I wrote: hobbies = customers .map(cust => cust.personal.map(person => person.hobbies)) .reduce((arr,hobby) => [...arr,...hobby], []);
Was I wrong to try to emulate his method here? I've seen that I could do this only using the reduce method, but I don't feel confident enough with reduce to know how. Help is much appreciated.