Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial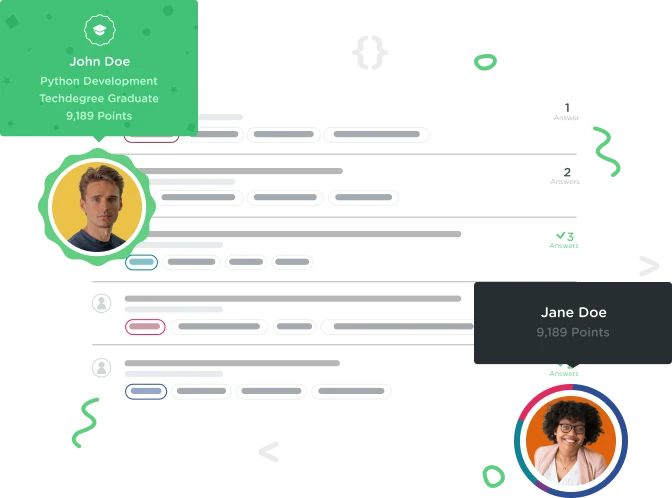
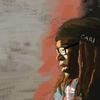
Carl Sergile
16,570 PointsArray/ for loop combo
Hey so not quite getting this concept. I have tried putting console.log in and outside of for loop. I don't understand how to get the for loop to display the temperatures the correct way.
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 101; i > 10; i -= 10) {
temperatures([i]);
console.log(temperatures);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
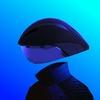
Kai Nightmode
37,045 PointsTry something like this...
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i++) {
console.log('The temperature at array position ' + i + ' is: ' + temperatures[i]);
}
We start at 0 and only loop as many times as the length of the temperatures array.
On each loop we write out what is at that position. For example, temperatures[0]
would be 100 and temperatures[1]
would be 90.
Try running the above code in your browser to see what happens. :D
Pedro Sousa
Python Development Techdegree Student 18,546 PointsHello Carl!
Array values are accessed using [ ] 's.
So, this is not correct, and you also don't need it.
temperatures([i]);
If you wanted to show the first value on the array, you would need to do something like:
console.log(temperatures[0]);
// this single line is getting the array named 'temperatures'
// accessing its first position
// and logging the result to the console. it will show '100'
So, what you need to do is have the console.log display the first element on the array (which is 100), then the second (90) all down to the last one.
First, will need to correct your 'for' header, the variable i will need to start on 0. Then, you will need to find out how to get maximum limit of i.
Then, inside the for loop, you'll need to use console.log almost as I used on the snipped above, BUT with a llliiiitle change :)
I really hope this helps!