Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial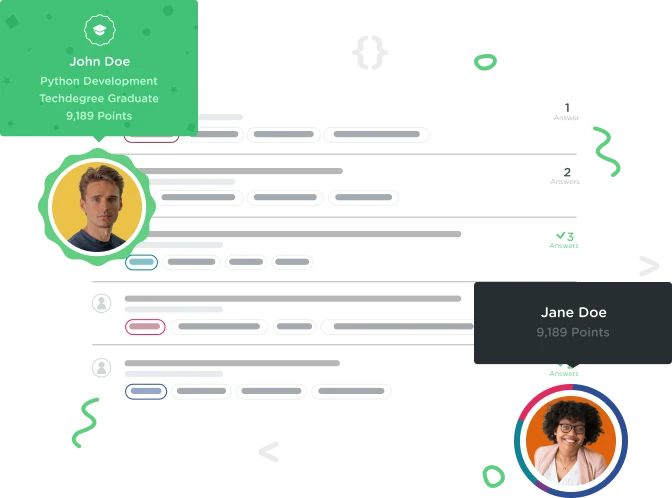

MIHA W.LEE
PHP Development Techdegree Graduate 25,452 PointsArray practice question
function createDeck() { var suites = ['♠︎','♣︎','♥︎','♦︎']; var ranks = ['Ace','King','Queen','Jack','10','9','8','7','6','5','4', '3','2']; var deck = []; // add your code below here: for (let i=0; i<suites.length; i++) { for (let j=0; j<ranks.length; j++) { let card = []; card.push(ranks[j], suites[i]); deck.push(card); } } return shuffle(deck); } // 6. Call the createDeck() function and store the results in a new variable named myDeck let myDeck = createDeck();
/* 7. Use a for loop to loop through the deck and list each card in the order the appear in the newly shuffled array. Use the log() method to print out a message like this, once for each card:
"7 of ♥.︎"
*/
for (let i = 0; i<myDeck.length; i++) {
console.log(myDeck[i][0]+ ' of ' + myDeck[i][1]);
}
Hi, First I dont understand the inner loop and outer loop, is it means when you run the first item in the outer loop, then it will load the rest of inner loop. When you load the second item in the outer loop, then it will load the rest of inner loop and so on. Is it true? Or inner loop first and outer loop second? Finally, there is shuffle algorithem i cant understand, can anyone explain to me detailed, thank you so much!
1 Answer
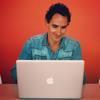
Remi Vledder
14,144 Points"Q1. I don't understand the inner loop and outer loop, is it means when you run the first item in the outer loop, then it will load the rest of inner loop. When you load the second item in the outer loop, then it will load the rest of inner loop and so on. Is it true? Or inner loop first and outer loop second?"
See comments in the code. It will run the code in the order it will find it. In this case the 'outer loop' first, and then runs all of the code in it once. In this case that is another loop. That loop will start to run and will create a card. And does that for all the cards that are in the ranks.
Once they all are done, the outer loop will start another iteration, namely the second iteration. And so fort.
Final result with comments:
function createDeck() {
var suites = ['♠︎','♣︎','♥︎','♦︎'];
var ranks = ['Ace','King','Queen','Jack','10','9','8','7','6','5','4', '3','2'];
var deck = [];
// Q1: It runs four times, each time for a suite
for (let indexSuites = 0; indexSuites < suites.length; indexSuites++) {
// Q1: each time, run the number of ranks (ranks.length) before returning to next iteration
for(let indexRanks = 0; indexRanks < ranks.length; indexRanks++) {
let card = [];
card.push(ranks[indexRanks]);
card.push(suites[indexSuites]);
// the deck is updated here each time a card is created
deck.push(card);
}
}
// The deck should only be returned once, therefore it's outside of the for loops
// Also, the deck should be shuffled:
return shuffle(deck);
}
const myDeck = createDeck();
for(let i = 0; i < myDeck.length; i++) {
const card = myDeck[i];
console.log(`${card[0]} of ${card[1]} `);
}
"Q2 Finally, there is shuffle algorithem i cant understand, can anyone explain to me detailed."
There is a source provided in the teachers notes for the shuffle algorithm: https://en.wikipedia.org/wiki/Fisher%E2%80%93Yates_shuffle
MIHA W.LEE
PHP Development Techdegree Graduate 25,452 PointsMIHA W.LEE
PHP Development Techdegree Graduate 25,452 PointsThank you so much!! I am almost understand it, but there are few things that i dont know, if i have question, i will ask you again.