Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial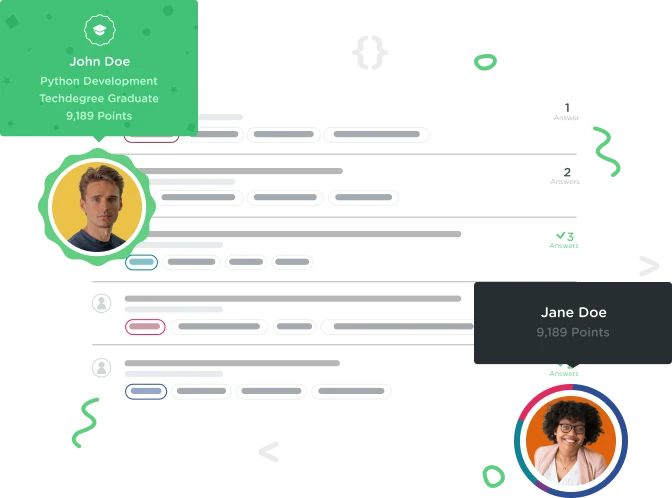

Philip Huffman
23,050 PointsArray should ONLY have one flavor?
I am very confused. The instructions say to add Avocado Chocolate to the array when it is not empty. However, if I do that a "Bummer!" message results. Would someone please point me in the right direction? Thank you.
<?php
$recommendations = array();
?><html>
<body>
<h1>Flavor Recommendations</h1>
<?php
if(!empty($recommendations)){
//unset $recommendations;
$recommendations = array('Avocado Chocolate');
echo '<ul>';
foreach($recommendations as $flavor) {
echo "<li> $flavor </li>";
}
echo '</ul>';
} else {
echo '<p>There are no flavor recommendations for you.</p>';
}
?>
</body>
</html>
1 Answer

Christian Andersson
8,712 Points<?php
$recommendations = array('Avocado Chocolate');
?>
You should add the element when you are initializing the array at the top of your code. What you are trying to do is a bit problematic because you are trying add the flavor inside an if
clause that will only be entered if the array was not empty to begin with -- so the flavor is never added!
In addition to this, you are trying to initialize your array a second time, which is not necessary. Only initialize variables once. The following doesn't apply in this particular problem (the correct code is above), but for future reference if you want to add an element to an array that has already been initialized you can use array_push
. Like this:
<?php
$numbersArray = array(); //this initializes the array
//code goes here
$a = 34;
array_push($numbersArray, $a);
//now the array has one element
$b = 50;
$c = 16;
array_push($numbersArray, $b, $c); //you can add multiple values at once if you want.
//now the array has 4 elements
$second_array = array(3, 7, 9);
array_push($numbersArray, $second_array); //you can also append an array to another!
//now the array has 7 elements
?>
http://php.net/manual/en/function.array-push.php
Hope this clears things up :)
Philip Huffman
23,050 PointsPhilip Huffman
23,050 PointsNever mind. I went back and reread the instructions. I feel so silly right now.