Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial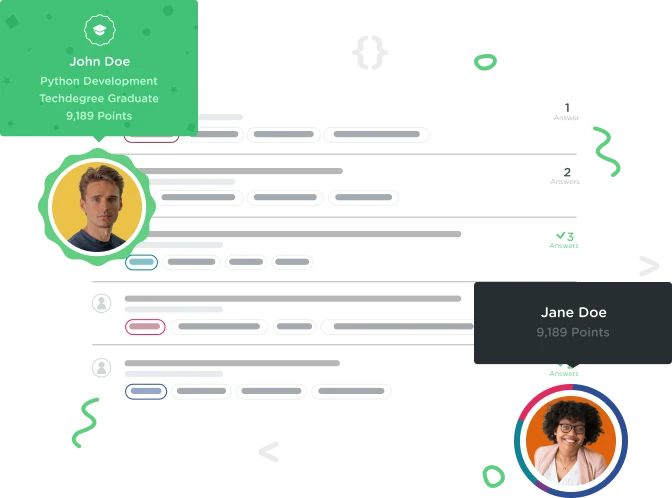

RODRIGO MARTINEZ
5,912 PointsArray with nested arrays of 3 numbers each representing hours, minutes, and seconds. Need to set all values to seconds
I have an array with nested arrays of 3 numbers each representing hours, minutes, and seconds respectively. Need to set all values to seconds
Ex: let numsArr = [[1, 15, 59], [1, 47, 6],[1, 32, 34], [2, 3, 17]];
In this example for instance the first nested array would mean 1 hour 15 min 59 sec. So i need to multiply 1 *3600 and 15 * 60 i guess. I just been going around and around with for loops and forEach loops but can't see how to make it. I've come all the way from having a string:
let strg = "01|15|59, 1|47|6, 01|17|20, 1|32|34, 2|3|17";
and transform it to an array with nested arrays of numbers. My final task is to get the average, mean and range of the given times. Not sure if my approach to sum in seconds is right.
let numsArr = [[1, 15, 59], [1, 47, 6],[1, 32, 34], [2, 3, 17]];
1 Answer
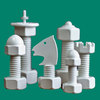
Steven Parker
229,732 PointsYou've got the right idea about multiplying, and you nearly had it using the nested array. For example, if you wanted to just show on the long the times in seconds for each group:
for (numbers of numsArr) {
let seconds = numbers[0] * 3600 + numbers[1] * 60 + numbers[2]; // convert to seconds
console.log(`[${numbers[0]},${numbers[1]},${numbers[2]}] is ${seconds} seconds`);
}
RODRIGO MARTINEZ
5,912 PointsRODRIGO MARTINEZ
5,912 PointsHello sensei MR. Parker. Thanks for helping out once again. I've tried the solution and i undestand the basics for how it works. However this gives me the sum result just for the first nested array. And what I think I 'd need to do is to sum the elements of each nested array.
That way i can order the results afterwards from the slowest time to the fastest time. And after ordering the times I'll be able to reconvert these results in seconds back to the "[hours, minutes, seconds]" format and then back to the string format of "house | minutes | seconds". I hope what i wrote makes sense to you. Sorry I'm still not proefficient with all this. Thanks again for taking the time.
RODRIGO MARTINEZ
5,912 PointsRODRIGO MARTINEZ
5,912 PointsOk. ignore previous message. I found it now
Steven Parker
229,732 PointsSteven Parker
229,732 PointsYou don't need that outer array, since the function returns during the first iteration:
And f all you want to do is sort them, you don't have to convert them — just sort first by hours, then by minutes (only if hours match), and then by seconds (if hours and minutes match).
The array.sort method takes a custom comparison function that would be useful to implementing this.
If your original question has been answered, you can mark the question solved by choosing a "best answer".
And happy coding!