Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial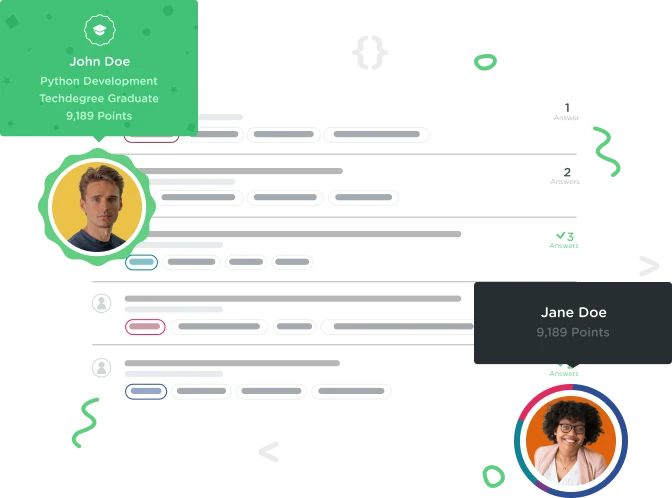

orange sky
Front End Web Development Techdegree Student 4,945 PointsarrayCounter, 'string', 'number', 'undefined'
Hello!
I am supposed the create a function that takes in an array, and the function must return the length of the items in the array.
I have to use an if() to check if any of the values in the array are either (string, number, undefined). I believe the task is to check If any of the values in the array are one of the 3, if I there is a match, I must return 0 to the console.
I have managed to get the length of the array, but I cant think of the right if () test to check for these values. I have always thought the only way to go through an array is with a for loop, but the assignments is expecting an if()
1) How can I conduct this test for these 3 values in the array?
2)When I do a console.log(typeof array);it returns 'Object', if the parameter is storing an array, shouldn't the web console say Array? Yes, I know arrays are objects, but I am new to using web console, so I just want to confirm. Thanks!! <!DOCTYPE html> <html lang="en"> <head> <title>JavaScript Foundations: Functions</title> <style> html { background: #FAFAFA; font-family: sans-serif; } </style> </head> <body> <h1>JavaScript Foundations</h1> <h2>Functions: Return Values</h2> <script>
function arrayCounter(array)
{
if ( typeof array === 'string' || 'number' || 'undefined')
{
return 0;
}
return array.length
}
console.log(arrayCounter(["apple", "oranges", "avocado"]));;
</script>
</body> </html>
3 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsYou are not supposed to check the elements of the array. An array is a single argument to this function. You have to check if that argument is in fact an array, or rather, you have to check that it's not a string, number or undefined.
And when checking multiple conditions in an if statement, you have to do each check separately:
function arrayCounter(array) {
if ( typeof array === 'string' || typeof array === 'number' || typeof array === 'undefined') { // you have to repeat the whole expression, not just the value you're comparing with
return 0;
}
return array.length;
}

orange sky
Front End Web Development Techdegree Student 4,945 PointsThanks Dino for your help. Just out of curiousity if I wanted to put some elements in my array and pass it to the arrayCounter so that the alert ('this is not a valid type') in the if() gets trigerred how can I do that? I tried with the code below, but .... Cheers!
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter(array)
{
if ( typeof array === 'string' || typeof array === 'number' || typeof array === 'undefined') { // you have to repeat the whole expression, not just the value you're comparing with
alert("It is an invalid type");
return 0;
}
return array.length;
}
console.log (arrayCounter(["apple", "orange"]));
</script>
</body>
</html>

Dino Paškvan
Courses Plus Student 44,108 PointsYour code will alert if you pass a parameter to the function that is not an array:
arrayCounter('A string');
// or
arrayCounter(123);

orange sky
Front End Web Development Techdegree Student 4,945 Pointshahaha! right!! and in that case, the alert should say ' it is a valid type'. Thanks a million Dino!