Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial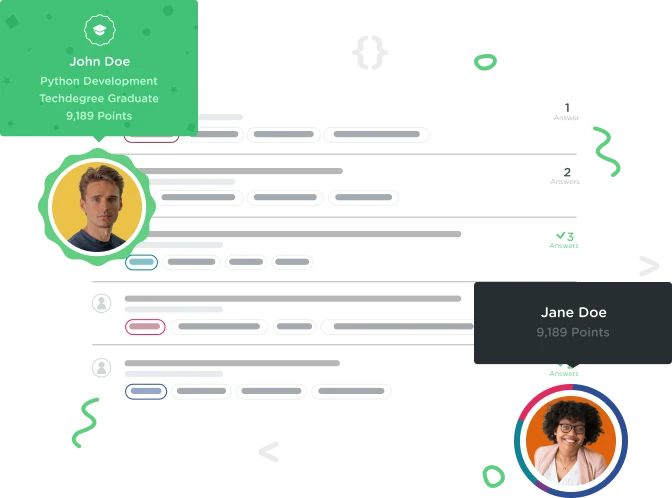
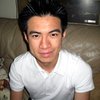
jason chan
31,009 PointsArrays and functions? what's happening inside the braces of the parenthesis?
var playList = [
'I Did It My Way',
'Respect',
'Imagine',
'Born to Run',
'Louie Louie',
'Maybellene'
];
function print(message) { // <---- what? where did message come from
document.write(message);
}
function printList(list) { // <---- what? where did list come from
var listHTML = '<ol>';
for (var i = 0; i < list.length; i += 1) {
listHTML += '<li>' + list[i] + '</li>'
}
listHTML += '</ol>';
print(listHTML);
}
printList(playList);
What's happening to message and list? I don't understand. The variables were not defined.
2 Answers

Chris Shaw
26,676 PointsHi Jason,
This can be a confusing concept for most new comers to languages such as JavaScript but fear not as it's very easy to understand, whenever we declare a function (in most languages, Ruby is slightly different along with some others), we can pass it arguments which as part of the declaration for that particular function.
Arguments example
var foo = 'Hello';
var bar = 'World';
someFunction(foo, bar); // foo and bar are the function arguments
Makes sense? If not don't worry as the concept of function arguments will come naturally as you progress and advance your JavaScript knowledge, so far you've seen how arguments work but what about function parameters?
What are parameters?
Parameters are very similar to arguments, in fact, they're one-in-the same. The common difference between the two is parameters are whatever we want them be called whereas an argument is a physical object we're passing into a function declaration.
Parameters can be named anything, in the case of the example code you have above message
is just a variable generated by the browser (it can be called anything such as valueOfSomeSort
) and can be used throughout the entire code execution of the function.
Parameter names as I mentioned can be called anything, once defined the browser takes care of declaring it within the scope (you can learn more about scopes on the Mozilla Developer Network) of the function. This variable can't be used outside of the function but will always contain a new value anytime the function is called.
function doSomething(foo, bar) {
// foo and bar don't require the "var" keyword because they are function parameters
}
Hope that helps.
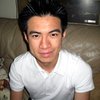
jason chan
31,009 Pointsso its' similar to var anything; It's like undefined variable. I think i got it.