Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial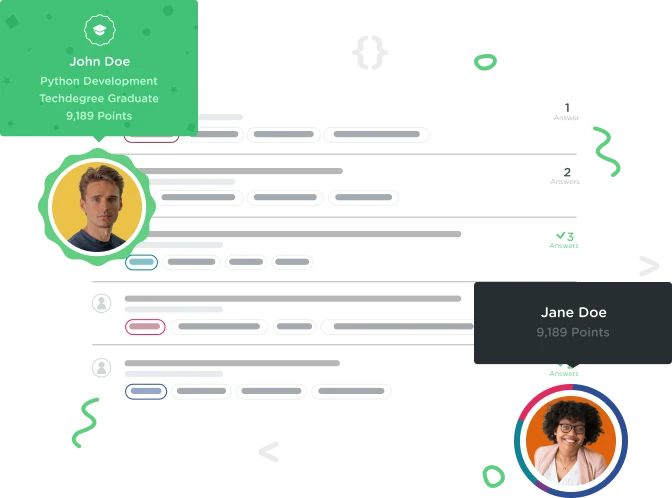

Steven Williams
2,199 PointsArrays.asList
Can someone please explain Arrays.asList? As I am watching the video, it is being used in this way: list<String> frontEndLanguages = Array.asList("HTML", "CSS", "Javascript");
Can someone explain to me what is happening here? Is Array.asList a method here? And what is list<String>? I get that String is a class and therefore it must be the type. True? But what does list mean here? Does that mean a list of Strings? Can both list and Array.asList be explained and please tie them together in the way they are being used here? Thanks.
2 Answers
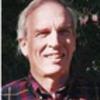
jcorum
71,830 PointsArrays.asList() turns an array into a list. Here's a sample program to demonstate:
import java.util.Arrays;
import java.util.List;
/*
* A java example source code to demonstrate
* the use of asList() method of Arrays class
*/
public class ArraysAsListExample {
public static void main(String[] args) {
// initialize a new String array
String[] array = new String[]{"A","B","C"};
// convert the student database into list
List alist = Arrays.asList(array);
// print how many student on the list
System.out.println("Count of letters: " + alist.size());
// print the contents of our list
System.out.println(alist);
// edit list
alist.set(2, "CC");
// print edited list
System.out.println(alist);
// print array
for (int i = 0; i < array.length; i++) {
System.out.print(array[i] + " ");
}
}
}
Not that changing the list also changes the array. (Sorry, the code indentation gets messed up when I paste it into the markup!).
Here's the output:
Count of letters: 3
[A, B, C]
[A, B, CC]
A B CC

Carlos Lázaro Costa
Courses Plus Student 1,615 PointsList<T>
is a lineal collection where you store elements of type T
(note that L is uppercase because is a class name). As you said, List<String>
means that the list is of String
objects. You can see the methods of Lists here.
Note that List is an interface, so you cannot instantiate it directly, but you can do it by some List implementations like ArrayList
or LinkedList
.
Arrays.asList(T... a)
converts an array of objects to a List<T>
.
Then, in your sentence
List<String> frontEndLanguages = Arrays.asList("HTML", "CSS", "Javascript");
The VM are doing the following steps:
- Initializes an
String[]
array of three positions with the elements in parameters. - Calls asList static method of class
Arrays
(notArray
class). - Result is assigned to the list named
frontEndLanguages
. More information of this method here.
Hope this answered your question. Greetings.

Steven Williams
2,199 PointsThank you!
Steven Williams
2,199 PointsSteven Williams
2,199 PointsPerfect. Thank you!