Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial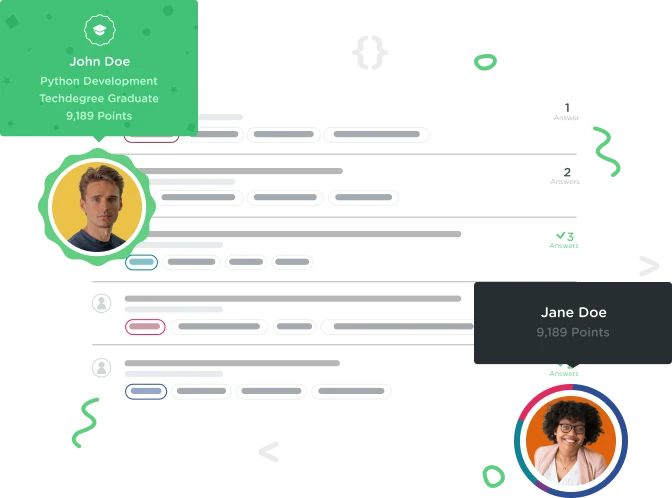
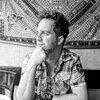
jlampstack
23,932 PointsArrow function binds the function to the instance....
At 2:20 in the video Guil says "Arrow function binds the function to the instance of the person no matter where it is called".
I understand this part, but can someone explain in simple terms, what was happening when we were using the original function() syntax?
1 Answer

Alexander La Bianca
15,959 PointsPrior to arrow functions, every new function defined its own 'this' value. so say you have the following class
//constructor
function Person() {
this.age = 0
//now in the constructor here create an interval that attempts to age the person every second
setInterval(function () {
//now you might think you can just access 'this' and think it is the person object.
//however, that is not true since you are in a new function, this will refer to the global object i belive
this.age++ //will not work
}, 1000)
}
//So if you now do
var person = new Person(); //You will likely get an undefined error as 'age' does not exist in the global object
//NOW THERE HAVE BEEN WAYS AROUND THIS BY REASINING 'this' INSIDE THE CONSTRUCTOR
//constructor
function Person() {
var self = this;
self.age = 0;
setInterval(function(){
//now you can access the 'self' variable in here which correctly refers to this as the Person
self.age++;
}, 1000);
}
var person = new Person(); // now should work and the person should age very second
//Now new with ES6 we can even skip this step
function Person() {
this.age = 0;
setInterval(()=>{
//since we are using arrow syntax, the this value will refer to person
this.age++;
},1000);
}
I hope that makes sense