Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial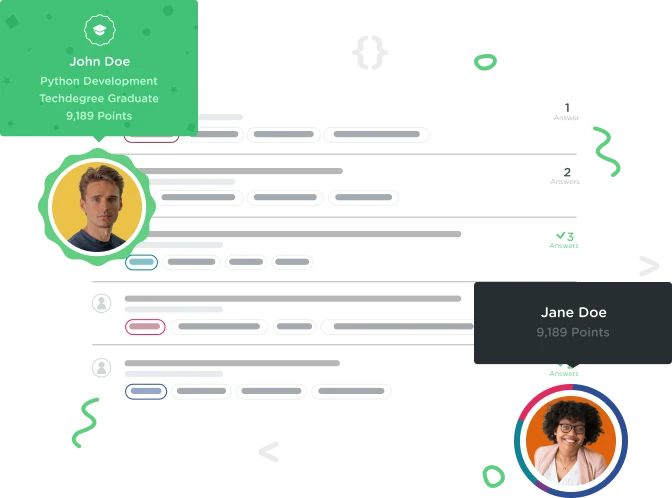
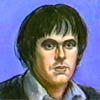
Jesse Thompson
10,684 PointsArrow function expression stopped code from working
So I learned about arrow function expressions so I decided to change my functions for the random quote generator. The first two functions getRandomNumber and getRandomQuote work fine when I change the functions to function expressions. But the 3rd one printQuote gives me an error "Uncaught ReferenceError: printQuote is not defined at script.js:3 (anonymous) @ script.js:3"
when I change it to const printQuote = () = > { etc...
Now when the printQuote function was typed out in the original way I didnt get this problem. I know I can just move that line of code to the end but Im wondering why this became an issue after I made the change? Seems really important when I'll be developing more complex applications.
```javascript // event listener to respond to "Show another quote" button clicks // when user clicks anywhere on the button, the "printQuote" function is called document.getElementById('loadQuote').addEventListener("click", printQuote, false);
// Array of quotes for random number generator. The random number generated will be used choose an index in the array to print. var quotesArray = [ {quote: "Let them be helpless like children, because weakness is a great thing, and strength is nothing" , source: "Andrei Tarkovsky", citation: "Stalker", year: "1979"}, {quote: "The man who lies to himself and listens to his own lie comes to a point that he cannot distinguish the truth within him, or around him, and so loses all respect for himself and for others. And having no respect he ceases to love." , source: "Fyodor Dostoyevsky", citation: "The Brothers Karamazov", year: "1880"}, {quote: "Do not give that which is holy to the dogs, nor cast your pearls before swine, lest they shall trample upon them with their feet, and having turned, tear you to pieces." , source: "Jesus Christ", citation: "The King James Bible", year: ""}, {quote: "And their taking usury though indeed they were forbidden it and their devouring the property of people falsely, and we have prepared for the unbelievers from among them a painful chastisement." , source: "Quran", citation: "", year: ""}, {quote: "What is compromise? Stupid. A man comes into my life and I have to compromise? You must think about that one again." , source: "Eartha Kitt", citation: "The Eartha Kitt Story", year: "1982"}, {quote: "You talkin' to me?" , source: "Travis Bickle", citation: "Taxi Driver", year: "1976"}, {quote: "Who looks outside, dreams. Who looks inside, awakens." , source: "Carl Jung", citation: "", year: ""}, {quote: "Where men are the most sure and arrogant, they are commonly the most mistaken, and have there given reins to passion, without that proper deliberation and suspense, which can alone secure them from the grossest absurdities." , source: "David Hume", citation: "", year: ""}, {quote: "Here's looking at you, kid." , source: "Rick", citation: "Casablanca", year: "1942"}, ];
var quoteHTML var printedQuotesArray = []; var chosenObject
// Logs array in console. for (var prop in quotesArray) { console.log(quotesArray[prop]); }
// Random number function to length of array. Rounds down to account for 0 index. const getRandomNumber = () => { var randomNumber = Math.floor(Math.random()* quotesArray.length); return randomNumber; }
// Logs random number 20 times to check functioning of getRandomNumber function. for (var i = 0; i < 20; i += 1) { console.log (getRandomNumber()); }
// Gets random quote based off of getRandomNumer() and builds HTML quote and details to print into HTML file. const getRandomQuote = () => { var i = getRandomNumber(); return quotesArray[i]; }
// Takes whatever array is returned from getRandomQuote() function, logs quotes in console and builds string into quoteHTML to print to index.html. const printQuote = () => { chosenObject = getRandomQuote(); quoteHTML = '<p class="quote">' + chosenObject.quote + '</p>'; quoteHTML += '<p class="source">' + chosenObject.source; if (chosenObject.citation !== "") { quoteHTML += '<span class="citation">' + chosenObject.citation + '</span>'; } if (chosenObject.year !== "") { quoteHTML += '<span class="year">' + chosenObject.year + '</span></p>'; } var quoteOutputDiv = document.getElementById('quote-box'); console.log(quoteHTML); quoteOutputDiv.innerHTML = quoteHTML; } printQuote(); // Automatically prints another quote every 30 seconds. setInterval(printQuote, 30000); ```
1 Answer
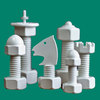
Steven Parker
231,198 PointsIt's not the arrow syntax but the fact that you also replaced function definitions with function expressions.
Function definitions are "hoisted", which means it's not important where they are placed in the code. But the assignment of a function expression must occur before that function is used or referenced.
Steven Parker
231,198 PointsSteven Parker
231,198 PointsIt looks like you tried to format your code, but those backticks must be on their own lines. The first set can be followed by the letters "js" or the word "javascript" to give syntax coloring.