Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial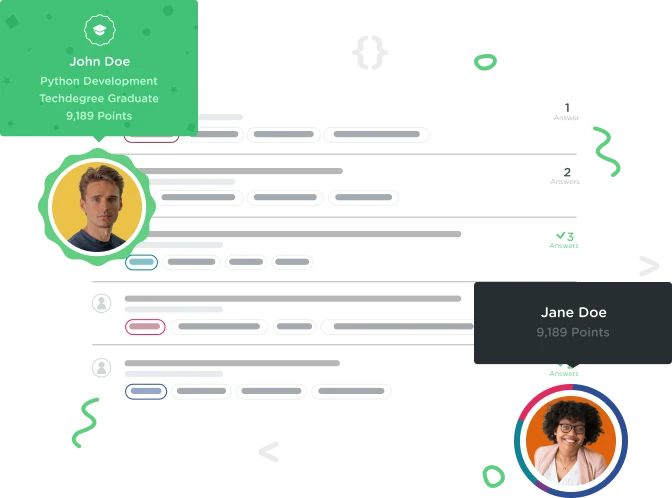

Frank keane
8,074 PointsArrow functions don't always use curly braces though.
I'm confused by the statement that arrow functions always use curly braces. A lot of what we've seen so far dont use braces users.filter( user => user.name !== "Samir");
is this because this is a declaration & not a logic function?
then the big leap to .map(name => ({ name })); can anyone explain why this is read as a declaration to create/add a property to the object?
Thanks!
2 Answers
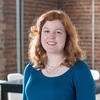
Sharon Hartsell
4,403 PointsI'll add on to Blake's great explanation in order to address your .map
question:
const users = userNames
.filter(name => user.name !== "Samir")
.map(name => ({ name }));
is the same as
const users = userNames
.filter(name => user.name !== "Samir")
.map(name => {
return {
name: name
};
});
The first code snippet is just using some shorthand syntax.
Shorthand Part 1: { name }
Creating an object property and setting it equal to a variable with the same name is pretty common, so JavaScript ES6 provides a shortcut to do that.
const dog = 'Woof';
// this...
const myPet = {
dog: dog
};
// { dog: 'Woof' }
// ...is the same as:
const yourPet = { dog };
// { dog: 'Woof' }
Shorthand Part 2: Parentheses ({ name })
The parentheses around { name }
tell the arrow function to return it as an object with the property name
. Without the parentheses, JS assumes you're just creating a function that doesn't do anything, like this
.map(name => {
name // yes, this is a variable, but we're not returning it, so it doesn't do anything
});
Hope this helps. Also, while syntax shortcuts are handy, I often choose to write out the longer version to make it easier for another developer to look at my code and quickly grasp what's going on. Code readability is more important than making the function happen in as few lines as possible.

Blake Larson
13,014 PointsWhen you have one line of code you can remove the curly braces and return
keyword because the arrow function will automatically know the one line is to be returned.
users.filter( user => user.name !== "Samir" );
is the same as
users.filter( user => { return user.name !== "Samir" } );

Frank keane
8,074 PointsThanks Blake!
Frank keane
8,074 PointsFrank keane
8,074 PointsBrilliant, thanks so much Sharon.