Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial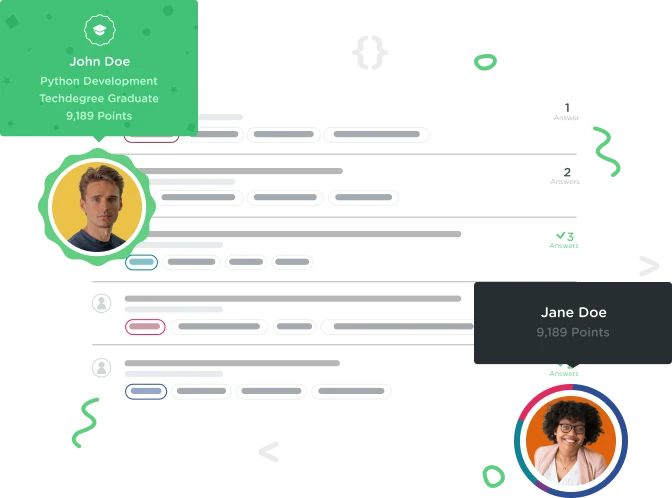
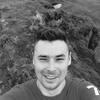
jamesjones21
9,260 PointsArrow functions within an object literal in JavaScript
Hi,
I have a question in relation to using arrow functions within an object literal.
The below code works as expected with the anonymous function being used
const ernie = {
//this is a key value pair where the animal property is representing the value of dog.
animal: 'dog',
age: 1,
breed: 'pug',
sentence: 'this is a test of words that we count',
//this is a method
bark: function () {
console.log('woof');
},
countWords: function() {
//to count words within a string we have to split the word by a space.
//cannot use arrow functions in a object literal
const splitWords = this.sentence.split(' ');
return splitWords.length;
}
}
console.log(ernie);
But the following code just gives an error when arrow functions are used to define a method within the object literal:
const ernie = {
//this is a key value pair where the animal property is representing the value of dog.
animal: 'dog',
age: 1,
breed: 'pug',
sentence: 'this is a test of words that we count',
//this is a method
bark: () => {
console.log('woof');
},
countWords: function() {
//to count words within a string we have to split the word by a space.
//cannot use arrow functions in a object literal
const splitWords = this.sentence.split(' ');
return splitWords.length;
}
}
console.log(ernie);
'''
Could someone please shed some light on this matter? :)

Rich Donnellan
Treehouse Moderator 27,696 PointsGlad to hear!
1 Answer

Rich Donnellan
Treehouse Moderator 27,696 PointsIn your second example, console.log(ernie)
isn't outside of the ernie
object literal.
It can't "log itself from within itself".
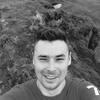
jamesjones21
9,260 PointsI understand that, but it kick's out an error whenever you try to use an arrow function to declare a function. Which is odd. The second console.log is a typo apologies

Rich Donnellan
Treehouse Moderator 27,696 PointsWhere are you trying to run this code? It works when I paste into Chrome DevTools.
jamesjones21
9,260 Pointsjamesjones21
9,260 PointsActually it does work -_- must have been me as it was late at night so thanks for the reply, just needed some extra sleep doh !