Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial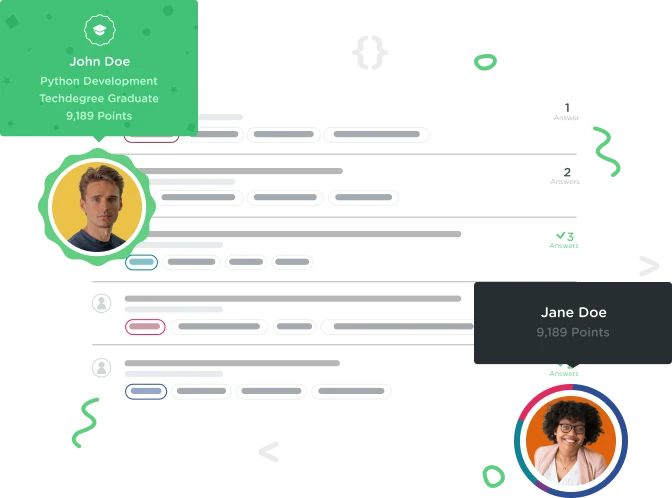

Andrew Mlamba
15,642 PointsArticle detail view - Challenge Task 3 of 3
Can't seem to pass Task 3. Here is my code.
from django.shortcuts import get_object_or_404, render
from django.http import Http404
from .models import Article, Writer
def article_list(request):
articles = Article.objects.all()
return render(request, 'articles/article_list.html', {'articles': articles})
def writer_detail(request, pk):
writer = Writer.objects.get(pk=pk)
return render(request, 'articles/writer_detail.html', {'writer': writer})
def article_detail(request, pk):
try:
article = Article.objects.get(pk=pk)
except Article.DoesNotExist:
raise Http404()
else:
return render(request, 'articles/article_detail.html', {'article': article})
from django.conf.urls import url, shortcuts
from . import views
urlpatterns = [
url(r'writer/(?P<pk>\d+)/$', views.writer_detail),
url(r'article/(?P<pk>\d+)/$', views.article_detail),
url(r'', views.article_list),
]
1 Answer
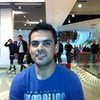
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Andrew
Task 3 is asking you to use the get_object_or_404.
def article_detail(request, pk):
article = get_object_or_404(Article, pk=pk)
return render(request, 'articles/article_detail.html', {'article': article})