Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial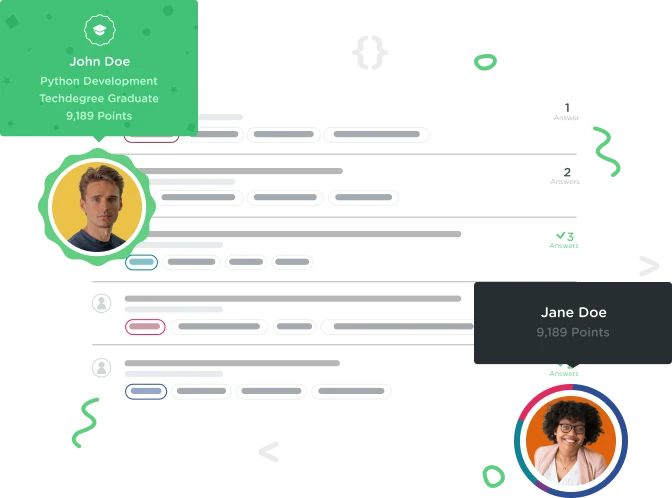
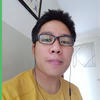
Rifqi Fahmi
23,164 Points[ASK] Can anyone explain to me this code ? (taken from MDN)
I got confused with this code and I always thinking about it and I still can't get it
var indices = [];
var array = ['a', 'b', 'a', 'c', 'a', 'd'];
var element = 'a';
var idx = array.indexOf(element);
while (idx != -1) {
indices.push(idx);
idx = array.indexOf(element, idx + 1);
}
console.log(indices);
// [0, 2, 4]
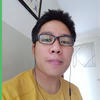
Rifqi Fahmi
23,164 Pointsthe
idx = array.indexOf(element, idx + 1);
and how can this loop stop?? what make it stop :')
5 Answers

Jacob Mishkin
23,117 PointsOkay, I could be wrong and if I am I hope someone corrects me, but this code is finding/logging all of the occurrences of the variable element or "a" in the array, and pushing its index into a new array. That is why it is logging 0,2,4.
To make the loop stop, look at the conditional statement of the while loop. it reads while idx which equals the array indexOf("a") or the variable element. Does NOT equal -1 which means while the element "a"'s index DOES exist in the in the array do something until this condition is false.
idx = array.indexOf(element, idx + 1);
here there is a couple of things going on:
while inside the while loop the variable idx gathers the index of the element variable which is "a" and then loops through itself by having idx +1 as the setter and ender of the search of the variable "a". While this is happing all the indices of the variable element which is "a" is pushed into a new array called indices. the condition is then false once all indices (index's) of the variable element which is "a" are pushed into the new array and out of the variable arry. This is why the indices logged 0,2,4.
I hope this helps. Let me know if you need me to break it down further.
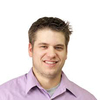
Kevin Korte
28,148 PointsYou're right Jacob. When indexOf finds the element to search for, which here is "a", it logs the index of that "a" into the indices, array, and than starts again, only this time searching from the next indexed array item so it doesn't count that same "a" twice.
The while loop finally stops when no more "a" 's are in the array, it will return -1. As long as there is an "a" somewhere in the array that the indexOf hasn't got to, it'll return that index instead. That's what keeps the while loop alive.
Upvote for you.

Jacob Mishkin
23,117 PointsThanks Kevin!
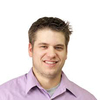
Kevin Korte
28,148 PointsWhen indexOf is given two arguments, the first is the search element, the second is fromIndex.
Your example, you're asking it to find 7, starting at index 5, but you don't have index 5, it doesn't exist. Index are zero based so what you really have is
var meBall = [ [0]=>2, [1]=>4, [2]=>5, [3]=>6, [4]=>7 ]
So because index 5 doesn't exist, it can't find the number 7, so it returns -1
If you changed your second argument to anything between 0 and 4, it will return 4, since index 4 is where it finds the number 7. You can also remove the second argument and it will search from the index 0 by default.
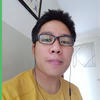
Rifqi Fahmi
23,164 Pointsthanks :)
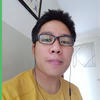
Rifqi Fahmi
23,164 Pointsnice got it, thanks :)

Jacob Mishkin
23,117 PointsNot a problem, Any Time!
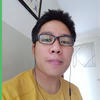
Rifqi Fahmi
23,164 Pointsjacob, how about this
var meBall = [2, 4, 5, 6, 7];
then when I type
meBall.indexOf(7, 5)
it returns -1 value. I don't understand how it work when indexOf() functio have more than 1 value to access the array such as .indexOf(4, 2), indexOf(6, 5), indexOf(4, 7), and so on.
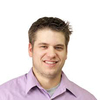
Kevin Korte
28,148 PointsIn regards, in plain english this is what you are asking with your examples using your array
- indexOf(7, 5) Look for integer 7, starting at index 5 of array Result: -1 (Index 5 doesn't exist)
- indexOf(4, 2) Look for integer 4, starting at number 5 (index 2) in array Result: -1 (4 not found in index 2, 3 or 4)
- indexOf(6, 5) Look for integer 6, starting at index 5 of array Result: -1 (Index 5 doesn't exist)
- indexOf(4, 7) Look for integer 4, starting at index 7 of array Result: -1 (Index 7 doesn't exist)

Jacob Mishkin
23,117 PointsIts, because of this.
string.indexOf(searchValue[, fromIndex])
Returns the index within the calling String object of the first occurrence of the specified value, starting the search at fromIndex, returns -1 if the value is not found.
Jacob Mishkin
23,117 PointsJacob Mishkin
23,117 Pointswhat part are you having problems with?