Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial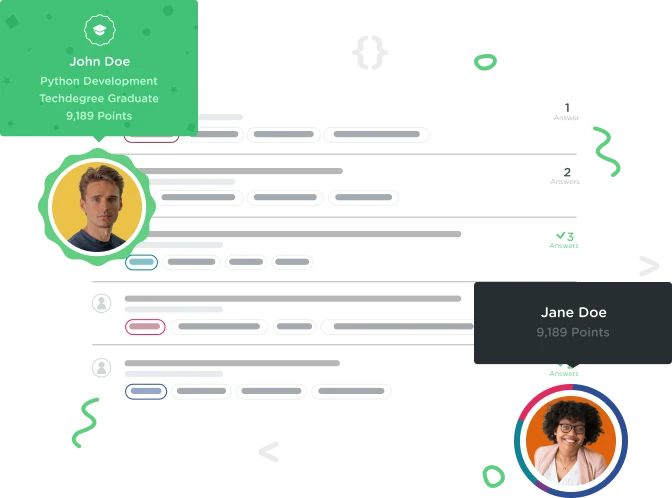

Gabor Kovacs
4,874 PointsAsk for help to my opposite game script
Hi! It's the main block of my script and I just need a little help. I don't know how to make it work so I would appreciate, if an expert give me a hand. Thanks in advance! :)
import random
my_num=int(input("My number: "))
def comp_guess():
a=1
b=10
guess = int(random.randint(a,b))
while True:
if guess == my_num:
print("You got it: ",my_num)
break
elif guess < my_num:
print("Comp number: {}".format(guess))
a+=1
instruction = input("Smaller/Bigger: ")
if instruction.lower() == "Bigger":
comp_guess()
elif guess > my_num:
print("comp number: {}".format(guess))
b+=1
instruction = input("Smaller/Bigger: ")
if instruction.lower() == "Smaller":
comp_guess()
comp_guess()
[MOD: fix formatting replaced ''' with ``` -cf]
3 Answers
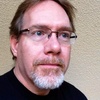
Chris Freeman
Treehouse Moderator 68,423 PointsI applaud the attempt at trying a recursive solution! It can sometime be difficult to clearly pass information from one recursive iteration to the next.
In each call to comp_guess()
, the variables a
and b
are unique to each recursive call. Hence these values are not changing between calls. You may want to change the function signature to def comp_guess(a=1, b=10):
then call the function with comp_guess(a, b)
.
The second issue is the instruction.lower()
comparisons to "Bigger" and "Smaller". These will never match since a lowered string will never have the capitalized "B" or "S".
Post back with your updated code if you need more help. Good Luck!!!

leakedmemory
11,304 PointsChris is definitely putting you on the right track. Also, when you program functionally and use recursion you won't need a loop.
Instead of printing the value, I would probably just return the number and pass that to a print statement.
You're really close, so don't give up.

Gabor Kovacs
4,874 PointsHi! First of all thanks for the advices!:) So here is my updated main script. It's working but it's not perfect. Sometimes it guess the same number. I try to avoid that with(just make a print for that so it's looks more pretty) the list option, but it isn't working. Any suggestion? Thanks in advance!
import random
my_num=(input("My number: "))
a = 1
b = 10
def comp_guess(a,b):
bad_guesses = []
guess = str((random.randint(a,b)))
if guess in bad_guesses:
print("You already guess this number{}.Try again!".format(guess))
comp_guess(a,b)
elif guess == my_num:
print("You got it: ",my_num)
print("Game Over")
elif guess < my_num:
a+=1
bad_guesses.append(guess)
print("Comp number: {}".format(guess))
instruction = input("Smaller/Bigger: ")
if instruction.lower() == "bigger":
print(" ")
comp_guess(a,b)
elif guess > my_num:
b-=1
bad_guesses.append(guess)
print("comp number: {}".format(guess))
instruction = input("Smaller/Bigger: ")
if instruction.lower() == "smaller":
print(" ")
comp_guess(a,b)
comp_guess(a,b)
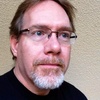
Chris Freeman
Treehouse Moderator 68,423 PointsLike a
and b
, the list bad_guesses
is local to each instance. It will also need to be passed to each recursive call.

Gabor Kovacs
4,874 PointsIt works perfectly now! Thank you!
import random
my_num=int((input("My number: ")))
a = 1
b = 10
bad_guesses = []
def comp_guess(a,b):
guess = (random.randint(a,b))
if guess in bad_guesses:
comp_guess(a,b)
elif guess == my_num:
print("You got it: ",my_num)
print("Game Over")
elif guess < my_num:
a = guess
bad_guesses.append(guess)
print("Comp number: {}".format(guess))
instruction = input("Smaller/Bigger: ")
if instruction.lower() == "bigger":
print(" ")
comp_guess(a,b)
else:
print("Bad instruction!")
elif guess > my_num:
b = guess
bad_guesses.append(guess)
print("comp number: {}".format(guess))
instruction = input("Smaller/Bigger: ")
if instruction.lower() == "smaller":
print(" ")
comp_guess(a,b)
else:
print("Bad instruction!")
comp_guess(a,b)