Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial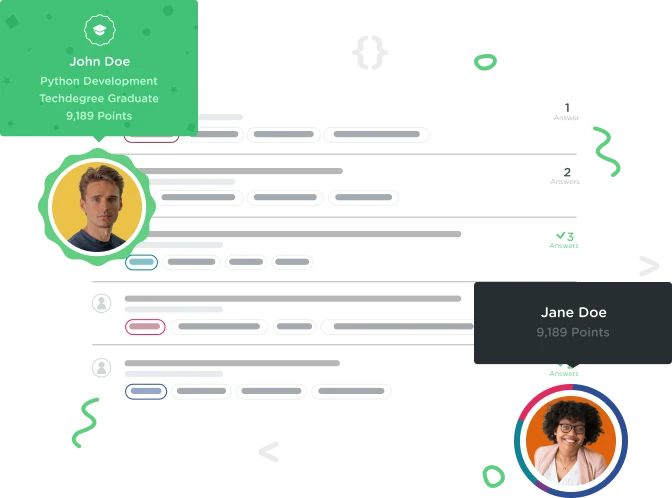

Yonas Mulyadi
8,000 PointsAsking for an answer regarding JavaScript DOM quiz
Hello guys, I got stuck in a DOM quiz. It says that I have to manipulate the colour of the list in HTML, and it appears working when I use this Js code. However, when I submit this code, it the bot says "Did you select all seven items?". Help me; I don't quite understand with this :). Thank you sincerely.
var listItems = document.getElementById ('rainbow');
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems.children[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
var listItems = document.getElementById ('rainbow');
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems.children[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
1 Answer

ada harrison
Full Stack JavaScript Techdegree Student 161 PointsFirst you have to select the ul with an ID #rainbow Then loop through the ul and its children with a for loop Apply the colors in the array to each children
const listItems = document.getElementById('rainbow'); //gets the <ul> with an ID #rainbow
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for (let i = 0; i < listItems.children.length; i++) { //loops through the <li> items which are children of <ul>
listItems.children[i].style.color = colors[i]; //applies the colors in the array to each children of the listitems as it loops
}
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsThe challenge wants you to update only update 1 line of code (
var listItems;
) with a method that will return all 7 list items. You are just returning 1 item and it is a UL element. So,getElementById()
is not the correct method.Can you find a method that will return all 7 list items?